vue springboot文件下载
时间: 2023-08-03 22:02:56 浏览: 109
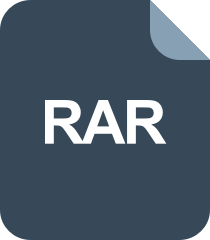
vue_springboot.rar
Spring Boot和Vue.js结合使用时,可以通过以下步骤实现文件下载:
1. 在Vue.js中,使用axios库发送请求到Spring Boot后端,获取文件的URL。
2. 在Spring Boot中,编写一个Controller方法,该方法接收文件名作为参数,并返回文件的字节数组。
3. 在Vue.js中,使用window.open方法打开文件的URL,浏览器会自动下载该文件。
下面是一个简单的示例代码:
Vue.js代码:
```
<template>
<div>
<button @click="downloadFile">下载文件</button>
</div>
</template>
<script>
import axios from 'axios'
export default {
methods: {
downloadFile() {
axios.get('/api/download?fileName=test.txt')
.then(response => {
const url = window.URL.createObjectURL(new Blob([response.data]))
const link = document.createElement('a')
link.href = url
link.setAttribute('download', 'test.txt')
document.body.appendChild(link)
link.click()
})
}
}
}
</script>
```
Spring Boot代码:
```
@RestController
@RequestMapping("/api")
public class FileController {
@GetMapping("/download")
public ResponseEntity<byte[]> downloadFile(@RequestParam String fileName) throws IOException {
File file = new File(fileName);
byte[] bytes = Files.readAllBytes(file.toPath());
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDisposition(ContentDisposition.attachment().filename(fileName).build());
return new ResponseEntity<>(bytes, headers, HttpStatus.OK);
}
}
```
在这个示例中,当用户点击“下载文件”按钮时,Vue.js会发送一个GET请求到Spring Boot后端的“/api/download”路径,并传递文件名“test.txt”作为参数。Spring Boot会读取该文件,并将其作为字节数组返回。Vue.js会使用window.URL.createObjectURL方法创建一个URL,然后使用document.createElement方法创建一个链接,将该URL设置为链接的href属性,将文件名设置为链接的download属性,最后将该链接添加到文档中并模拟点击该链接,浏览器会自动下载该文件。
阅读全文
相关推荐
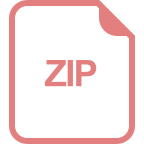
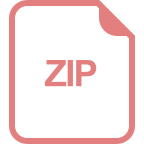
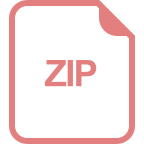
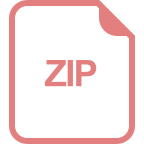
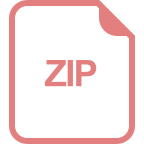
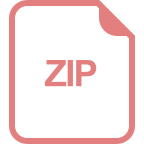
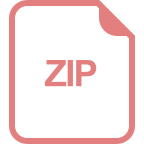
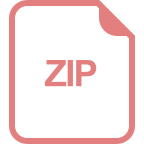
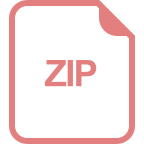
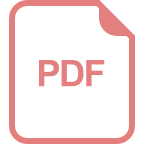
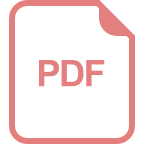
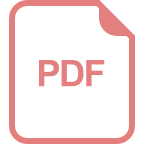
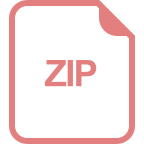
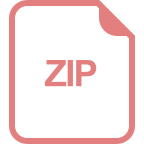