用springboot+vue+mybatis写一个文件上传功能
时间: 2023-05-17 17:02:35 浏览: 152
好的,这个问题我可以回答。首先,你需要在前端页面中添加一个文件上传的表单,然后使用Vue.js来处理表单的提交事件。在后端,你可以使用Spring Boot来处理文件上传的请求,并使用MyBatis来将文件信息存储到数据库中。
以下是一个简单的示例代码:
前端代码:
```
<template>
<div>
<form @submit.prevent="submitForm">
<input type="file" ref="fileInput" />
<button type="submit">上传文件</button>
</form>
</div>
</template>
<script>
export default {
methods: {
submitForm() {
const formData = new FormData();
formData.append('file', this.$refs.fileInput.files[0]);
// 发送文件上传请求
axios.post('/api/upload', formData).then(response => {
console.log(response.data);
});
}
}
};
</script>
```
后端代码:
```
@RestController
@RequestMapping("/api")
public class FileUploadController {
@Autowired
private FileService fileService;
@PostMapping("/upload")
public ResponseEntity<?> uploadFile(@RequestParam("file") MultipartFile file) {
try {
// 将文件保存到本地
String fileName = fileService.saveFile(file);
// 将文件信息存储到数据库中
fileService.saveFileInfo(fileName, file.getOriginalFilename(), file.getSize());
return ResponseEntity.ok().body("文件上传成功");
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("文件上传失败");
}
}
}
@Service
public class FileServiceImpl implements FileService {
@Autowired
private FileMapper fileMapper;
@Override
public String saveFile(MultipartFile file) throws IOException {
String fileName = UUID.randomUUID().toString() + "-" + file.getOriginalFilename();
Path filePath = Paths.get("uploads", fileName);
Files.copy(file.getInputStream(), filePath, StandardCopyOption.REPLACE_EXISTING);
return fileName;
}
@Override
public void saveFileInfo(String fileName, String originalFileName, long fileSize) {
FileEntity fileEntity = new FileEntity();
fileEntity.setFileName(fileName);
fileEntity.setOriginalFileName(originalFileName);
fileEntity.setFileSize(fileSize);
fileMapper.insert(fileEntity);
}
}
@Mapper
public interface FileMapper {
@Insert("INSERT INTO file (file_name, original_file_name, file_size) VALUES (#{fileName}, #{originalFileName}, #{fileSize})")
void insert(FileEntity fileEntity);
}
public interface FileService {
String saveFile(MultipartFile file) throws IOException;
void saveFileInfo(String fileName, String originalFileName, long fileSize);
}
public class FileEntity {
private int id;
private String fileName;
private String originalFileName;
private long fileSize;
// 省略 getter 和 setter 方法
}
```
这个示例代码中,我们使用了Vue.js来创建一个简单的文件上传表单,并使用axios库来发送文件上传请求。在后端,我们使用了Spring Boot来处理文件上传请求,并使用MyBatis来将文件信息存储到数据库中。
阅读全文
相关推荐
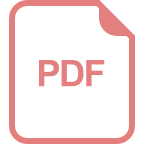
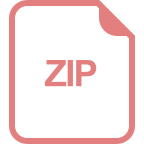
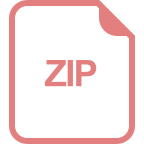
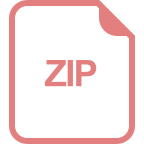
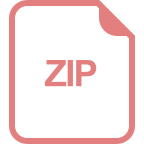
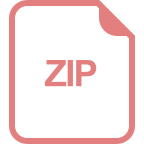
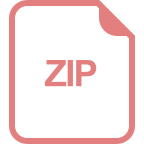
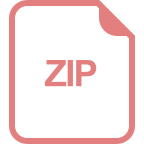
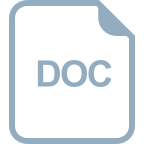



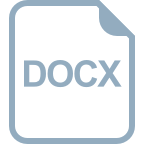
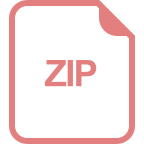
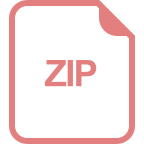
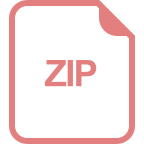
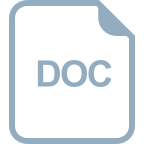
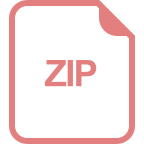