两个线程调度不均匀如何处理 demo
时间: 2023-08-22 22:08:35 浏览: 48
以下是一个简单的示例,演示如何使用计时器和中断机制来实现线程的时间片轮转调度,从而避免线程的调度不均匀问题。
```python
import threading
import time
# 定义一个全局变量,用于记录线程的执行顺序
g_count = 0
# 定义一个线程类
class MyThread(threading.Thread):
def __init__(self, name):
super().__init__()
self.name = name
def run(self):
global g_count
for i in range(10):
# 用锁来保证线程安全
with threading.Lock():
print(f"{self.name}: count={g_count}")
g_count += 1
# 让线程休眠一段时间,模拟线程的执行时间
time.sleep(0.1)
# 定义一个定时器类
class MyTimer:
def __init__(self, interval):
self.interval = interval
self.timer = None
self.is_running = False
def _run(self):
global g_count
while self.is_running:
# 每隔一段时间触发一次定时器中断
time.sleep(self.interval)
# 用锁来保证线程安全
with threading.Lock():
print(f"Timer: count={g_count}")
g_count += 1
def start(self):
self.is_running = True
self.timer = threading.Thread(target=self._run)
self.timer.start()
def stop(self):
self.is_running = False
if self.timer:
self.timer.join()
# 创建两个线程
thread1 = MyThread("Thread1")
thread2 = MyThread("Thread2")
# 创建一个定时器,每500毫秒触发一次中断
timer = MyTimer(0.5)
# 启动线程和定时器
thread1.start()
thread2.start()
timer.start()
# 等待线程和定时器结束
thread1.join()
thread2.join()
timer.stop()
```
在上面的示例中,我们创建了两个线程 `thread1` 和 `thread2`,并且创建了一个定时器 `timer`。定时器每隔500毫秒触发一次中断,从而触发线程的调度。每个线程执行10次,每次执行时都会输出一个计数器 `g_count` 的值。在输出计数器的值时,我们使用了一个线程锁来保证线程安全。在每个线程执行一段时间后,会调用 `time.sleep()` 方法来模拟线程的执行时间,从而让线程有机会被中断和调度。
通过运行上面的示例,我们可以看到两个线程的执行顺序是交替进行的,并且定时器也会定期触发中断,从而保证每个线程都有机会被调度执行。这样就可以避免线程的调度不均匀问题。
相关推荐
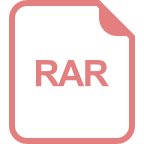














