import java.awt.BorderLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JTextField; public class PingProgram extends JFrame implements ActionListener { private static final long serialVersionUID = 1L; private JTextField ipAddressField; private JLabel resultLabel; public PingProgram() { setTitle("Ping Program"); setSize(400, 150); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); ipAddressField = new JTextField(20); JLabel ipAddressLabel = new JLabel("Enter IP Address: "); JPanel panel1 = new JPanel(); panel1.add(ipAddressLabel); panel1.add(ipAddressField); JButton pingButton = new JButton("Ping"); pingButton.addActionListener(this); JPanel panel2 = new JPanel(); panel2.add(pingButton); resultLabel = new JLabel(); JPanel panel3 = new JPanel(); panel3.add(resultLabel); add(panel1, BorderLayout.NORTH); add(panel2, BorderLayout.CENTER); add(panel3, BorderLayout.SOUTH); setVisible(true); } public void actionPerformed(ActionEvent e) { String ipAddress = ipAddressField.getText(); try { Process process = Runtime.getRuntime().exec("ping " + ipAddress); BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream())); String line; String result = ""; while ((line = reader.readLine()) != null) { result += line + "\n"; } resultLabel.setText(result); } catch (IOException ex) { resultLabel.setText("Error: " + ex.getMessage()); } } public static void main(String[] args) { new PingProgram(); } }能帮我把这段代码的界面内容一下分行吗
时间: 2024-04-28 12:26:34 浏览: 13
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class PingProgram extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JTextField ipAddressField;
private JLabel resultLabel;
public PingProgram() {
setTitle("Ping Program");
setSize(400, 150);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
ipAddressField = new JTextField(20);
JLabel ipAddressLabel = new JLabel("Enter IP Address: ");
JPanel panel1 = new JPanel();
panel1.add(ipAddressLabel);
panel1.add(ipAddressField);
JButton pingButton = new JButton("Ping");
pingButton.addActionListener(this);
JPanel panel2 = new JPanel();
panel2.add(pingButton);
resultLabel = new JLabel();
JPanel panel3 = new JPanel();
panel3.add(resultLabel);
add(panel1, BorderLayout.NORTH);
add(panel2, BorderLayout.CENTER);
add(panel3, BorderLayout.SOUTH);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
String ipAddress = ipAddressField.getText();
try {
Process process = Runtime.getRuntime().exec("ping " + ipAddress);
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
String result = "";
while ((line = reader.readLine()) != null) {
result += line + "\n";
}
resultLabel.setText(result);
} catch (IOException ex) {
resultLabel.setText("Error: " + ex.getMessage());
}
}
public static void main(String[] args) {
new PingProgram();
}
}
相关推荐
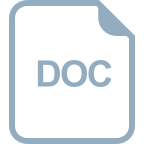
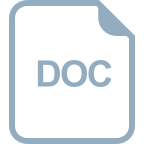
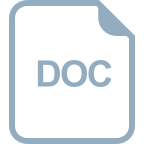















