读取一个文件,打印除了以#开头的行之外的所有行。文件名为file.txt.用函数封装
时间: 2023-11-27 18:50:09 浏览: 160
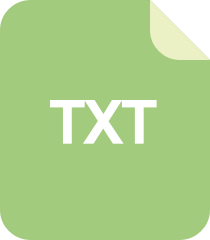
c# 读取txt文件
可以使用以下代码实现:
```python
def print_file_without_comments(file_name):
with open(file_name, 'r') as f:
for line in f:
if not line.startswith('#'):
print(line.strip())
```
这个函数打开指定的文件,逐行读取文件内容,并忽略以 # 开头的行,打印其他行。`strip()` 方法用于删除行末的空白字符。你可以将文件名作为参数传递给这个函数,如下所示:
```python
print_file_without_comments('file.txt')
```
这将打印出 `file.txt` 文件中除以 # 开头的行之外的所有行。
阅读全文
相关推荐
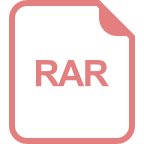
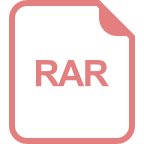
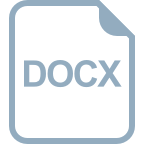
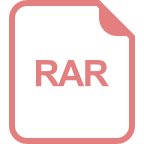
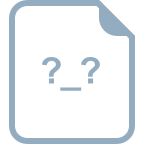
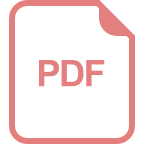
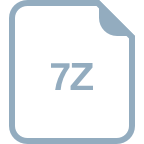
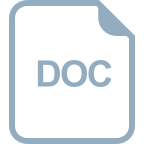
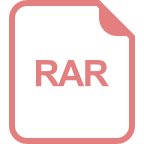
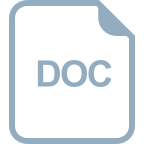
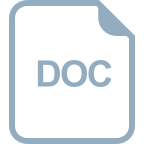
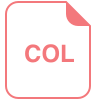
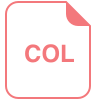
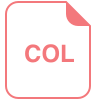
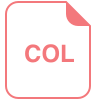
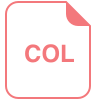