QLabel播放MP4
时间: 2024-01-11 19:05:39 浏览: 105
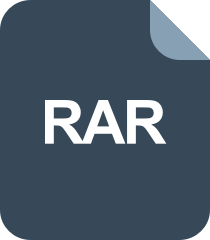
Qt实现在QLabel上播放视频文件,可以拖动进度条,快进倒退
QLabel不支持播放MP4文件,需要使用Qt的多媒体框架QMediaPlayer和QVideoWidget来实现MP4文件的播放。具体实现如下:
1. 创建一个QMediaPlayer对象并设置MP4文件路径:
```python
self.mediaPlayer = QMediaPlayer()
self.mediaPlayer.setMedia(QUrl.fromLocalFile("path/to/your/mp4/file"))
```
2. 创建一个QVideoWidget对象用于显示视频画面:
```python
self.videoWidget = QVideoWidget()
self.mediaPlayer.setVideoOutput(self.videoWidget)
```
3. 在需要播放视频的地方调用QMediaPlayer的play()方法:
```python
self.mediaPlayer.play()
```
4. 将QVideoWidget添加到QLabel中显示:
```python
self.label.setCentralWidget(self.videoWidget)
```
完整代码示例:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QVBoxLayout
from PyQt5.QtMultimedia import QMediaPlayer, QMediaContent
from PyQt5.QtMultimediaWidgets import QVideoWidget
from PyQt5.QtCore import QUrl
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('MP4 Player')
# create QMediaPlayer and set MP4 file path
self.mediaPlayer = QMediaPlayer()
self.mediaPlayer.setMedia(QMediaContent(QUrl.fromLocalFile("path/to/your/mp4/file")))
# create QVideoWidget to display video
self.videoWidget = QVideoWidget()
self.mediaPlayer.setVideoOutput(self.videoWidget)
# create QLabel to contain QVideoWidget
self.label = QLabel()
layout = QVBoxLayout(self.label)
layout.addWidget(self.videoWidget)
self.setCentralWidget(self.label)
# play video
self.mediaPlayer.play()
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
注意:需要安装Qt的多媒体模块才能使用QMediaPlayer和QVideoWidget。在PyQt5中使用时,需要在安装PyQt5时选择包含多媒体模块。如果没有选择,则需要重新安装PyQt5并选择包含多媒体模块。
阅读全文
相关推荐
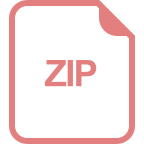
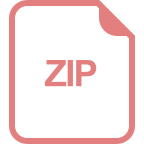
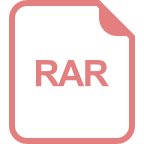











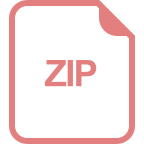
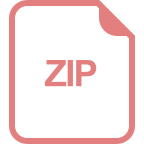
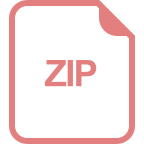