vhdl实现四人表决器
时间: 2023-09-28 18:04:14 浏览: 183
四人表决器是一种常见的电路,在数字电路中有很多应用。在VHDL中实现四人表决器可以采用状态机的方式进行。下面是一个简单的四人表决器的VHDL代码:
```
library ieee;
use ieee.std_logic_1164.all;
entity four_bit_voter is
port(
a : in std_logic;
b : in std_logic;
c : in std_logic;
d : in std_logic;
output : out std_logic
);
end entity four_bit_voter;
architecture Behavioral of four_bit_voter is
type state_type is (s0, s1, s2, s3, s4);
signal current_state, next_state : state_type;
begin
-- 状态转移逻辑
process(current_state, a, b, c, d)
begin
case current_state is
when s0 =>
if a = '1' then next_state <= s1;
else next_state <= s0;
end if;
when s1 =>
if b = '1' then next_state <= s2;
else next_state <= s1;
end if;
when s2 =>
if c = '1' then next_state <= s3;
else next_state <= s2;
end if;
when s3 =>
if d = '1' then next_state <= s4;
else next_state <= s3;
end if;
when s4 =>
next_state <= s4;
end case;
end process;
-- 输出逻辑
process(current_state)
begin
case current_state is
when s0 =>
output <= '0';
when s1 =>
output <= '0';
when s2 =>
output <= '1';
when s3 =>
output <= '1';
when s4 =>
output <= '1';
end case;
end process;
-- 状态更新
process(next_state)
begin
current_state <= next_state;
end process;
end architecture Behavioral;
```
在上面的代码中,我们定义了一个五种状态的状态机,每个状态代表了四个输入的某种组合情况。在状态转移逻辑中,我们通过判断当前状态和输入的值来计算出下一个状态。在输出逻辑中,根据当前状态来输出最终的结果。最后,在状态更新逻辑中,我们将当前状态更新为下一个状态。
阅读全文
相关推荐
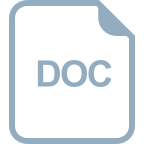
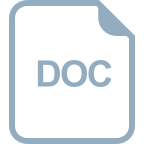
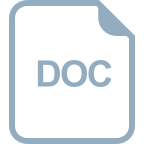
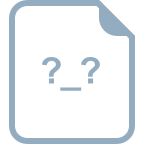
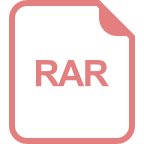
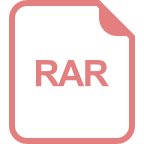

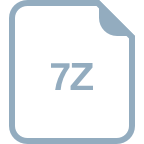
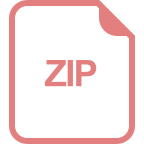
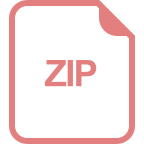
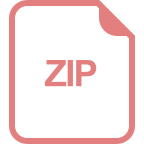
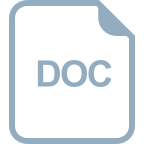
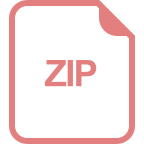
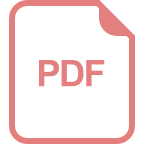
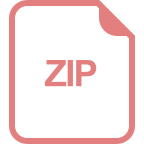
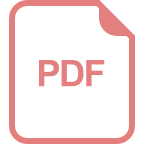
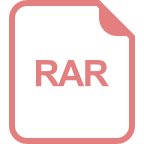
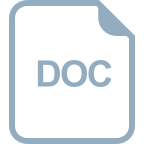
