请解释: //创建socket user.setUsername(username); socket = new Socket(InetAddress.getByName(ipText.getText()), Integer.parseInt(portText.getText())); //得到ObjectOutputStream ObjectOutputStream oos = new ObjectOutputStream(socket.getOutputStream()); //发送user对象 oos.writeObject(user); //读取服务端回复 ObjectInputStream ois = new ObjectInputStream(socket.getInputStream()); Message message = (Message) ois.readObject(); //判断是否登录成功
时间: 2024-02-14 11:18:58 浏览: 100
这段代码实现了一个客户端向服务器发送用户登录信息的功能,具体实现步骤如下:
1. 创建一个 Socket 对象,该对象表示客户端与服务器的通信管道。
2. 从客户端界面中获取用户输入的 IP 地址和端口号,使用 InetAddress 类的 getByName() 方法将 IP 地址转换为 InetAddress 对象。
3. 创建一个 ObjectOutputStream 对象,该对象封装了 Socket 的输出流,并用于将 Java 对象序列化后发送到服务器。
4. 将用户登录信息对象 user 序列化后发送给服务器,使用 writeObject() 方法将序列化后的数据发送到服务器。
5. 创建一个 ObjectInputStream 对象,该对象封装了 Socket 的输入流,并用于读取服务器返回的 Java 对象。
6. 从 ObjectInputStream 中读取服务器返回的 Message 对象,使用 readObject() 方法将对象反序列化。
7. 对 Message 对象进行解析,判断用户登录是否成功。
总的来说,这段代码实现了客户端与服务器之间的网络通信,并实现了用户登录功能。
相关问题
我的配置文件ebean: currentUserProvider: com.houdu.template.admin.common.context.CurrentUser logging: directory: logs iud: sql logfilesharing: all query: sql sqlquery: sql # txnCommit: none datasource: db: username: root password: fs@admin url: jdbc:mysql://192.168.0.248:3306/yitiji?useUnicode=true&characterEncoding=utf8&zeroDateTimeBehavior=convertToNull&useSSL=true&serverTimezone=GMT%2B8 driver: com.mysql.cj.jdbc.Driver和@Configuration @PropertySource("classpath:application.yaml") public class EbeanConfig { @Value("${datasource.db.url}") private String url; @Value("${datasource.db.username}") private String username; @Value("${datasource.db.password}") private String password; @Value("${datasource.db.driver}") private String driver; @PostConstruct public void init() { DataSourceConfig dataSourceConfig = new DataSourceConfig(); dataSourceConfig.setUsername(username); dataSourceConfig.setPassword(password); dataSourceConfig.setUrl(url); dataSourceConfig.setDriver(driver); DatabaseConfig config = new DatabaseConfig(); config.setName("db"); config.setDefaultServer(true); config.setDataSourceConfig(dataSourceConfig); config.setCurrentUserProvider(new CurrentUser()); DatabaseFactory.create(config); } } 这样的,还有什么问题吗
根据你提供的配置文件和代码,可能的问题是你在`EbeanConfig`类中使用了错误的注解`@PropertySource("classpath:application.yaml")`,它应该是`@PropertySource("classpath:application.properties")`,因为你的配置文件是`.properties`格式而不是`.yaml`格式。
请将注解修正为`@PropertySource("classpath:application.properties")`,然后重新运行应用程序,看看问题是否解决了。如果问题仍然存在,请提供详细的错误信息和相关代码,以便更好地帮助你解决问题。
Drawer::Drawer(QWidget *parent, Qt::WindowFlags f) :QToolBox(parent, f) { setWindowTitle(tr("Myself QQ 2013")); //设置主窗口的标题 setWindowIcon(QPixmap(":/image/qq.png")); //设置主窗体标题栏图标 toolBtn1 = new QToolButton; toolBtn1->setText(tr("道枝小尧")); toolBtn1->setIcon(QPixmap(":/image/spqy.png")); toolBtn1->setIconSize(QPixmap(":/image/spqy.png").size()); toolBtn1->setAutoRaise(true); toolBtn1->setToolButtonStyle(Qt::ToolButtonTextBesideIcon); connect(toolBtn1, SIGNAL(clicked()), this, SLOT(showChatWidget1())); toolBtn2 = new QToolButton; toolBtn2->setText(tr("忆梦如澜")); toolBtn2->setIcon(QPixmap(":/image/ymrl.png")); toolBtn2->setIconSize(QPixmap(":/image/ymrl.png").size()); toolBtn2->setAutoRaise(true); toolBtn2->setToolButtonStyle(Qt::ToolButtonTextBesideIcon); connect(toolBtn2, SIGNAL(clicked()), this, SLOT(showChatWidget2())); toolBtn3 = new QToolButton; toolBtn3->setText(tr("出版人")); toolBtn3->setIcon(QPixmap(":/image/qq.png")); toolBtn3->setIconSize(QPixmap(":/image/qq.png").size()); toolBtn3->setAutoRaise(true); toolBtn3->setToolButtonStyle(Qt::ToolButtonTextBesideIcon); connect(toolBtn3, SIGNAL(clicked()), this, SLOT(showChatWidget3())); toolBtn4 = new QToolButton; toolBtn4->setText(tr("Cherry")); toolBtn4->setIcon(QPixmap(":/image/Cherry.png")); toolBtn4->setIconSize(QPixmap(":/image/Cherry.png").size()); toolBtn4->setAutoRaise(true); toolBtn4->setToolButtonStyle(Qt::ToolButtonTextBesideIcon); connect(toolBtn4, SIGNAL(clicked()), this, SLOT(showChatWidget4())); toolBtn5 = new QToolButton; toolBtn5->setText(tr("淡然")); toolBtn5->setIcon(QPixmap(":/image/dr.png")); toolBtn5->setIconSize(QPixmap(":/image/dr.png").size()); toolBtn5->setAutoRaise(true); toolBtn5->setToolButtonStyle(Qt::ToolButtonTextBesideIcon); connect(toolBtn5, SIGNAL(clicked()), this, SLOT(showChatWidget5())); toolBtn6 = new QToolButton; toolBtn6->setText(tr("娇娇")); toolBtn6->setIcon(QPixmap(":/image/jj.png")); toolBtn6->setIconSize(QPixmap(":/image/jj.png").size()); toolBtn6->setAutoRaise(true); toolBtn6->setToolButtonStyle(Qt::ToolButtonTextBesideIcon); connect(toolBtn6, SIGNAL(clicked()), this, SLOT(showChatWidget6())); toolBtn7 = new QToolButton; toolBtn7->setText(tr("洛水无痕")); toolBtn7->setIcon(QPixmap(":/image/lswh.png")); toolBtn7->setIconSize(QPixmap(":/image/lswh.png").size()); toolBtn7->setAutoRaise(true); toolBtn7->setToolButtonStyle(Qt::ToolButtonTextBesideIcon); connect(toolBtn7, SIGNAL(clicked()), this, SLOT(showChatWidget7()));改为连接网络数据库
将以上代码改为连接网络数据库需要进行以下步骤:
1. 首先需要创建一个数据库,并在其中创建一个名为“users”的表,包含“id”、“name”和“avatar”三个字段,分别代表用户的ID、昵称和头像路径。
2. 然后需要使用Qt提供的QSqlDatabase类来连接数据库,可以使用以下代码:
QSqlDatabase db = QSqlDatabase::addDatabase("QMYSQL");
db.setHostName("localhost"); //数据库服务器地址
db.setPort(3306); //数据库端口号
db.setDatabaseName("mydatabase"); //数据库名称
db.setUserName("root"); //数据库用户名
db.setPassword("123456"); //数据库密码
bool ok = db.open();
if (ok) {
qDebug() << "数据库连接成功!";
} else {
qDebug() << "数据库连接失败!";
}
3. 连接成功后,可以通过QSqlQuery类来执行SQL语句,获取用户信息并显示在界面上。以下是示例代码:
QSqlQuery query;
query.exec("SELECT * FROM users");
while (query.next()) {
QString name = query.value("name").toString();
QString avatar = query.value("avatar").toString();
QToolButton *btn = new QToolButton;
btn->setText(name);
btn->setIcon(QPixmap(avatar));
btn->setIconSize(QPixmap(avatar).size());
btn->setAutoRaise(true);
btn->setToolButtonStyle(Qt::ToolButtonTextBesideIcon);
connect(btn, SIGNAL(clicked()), this, SLOT(showChatWidget()));
//将按钮添加到界面上
this->addItem(btn, "好友列表");
}
阅读全文
相关推荐
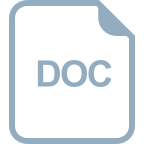
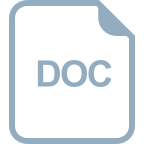
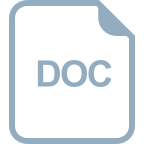












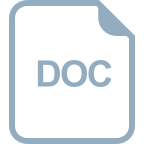
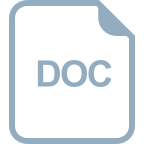