python中将两个列表的元素,从第n位后全部交换怎么写代码
时间: 2023-06-14 09:04:52 浏览: 58
可以使用切片和列表拼接来实现:
```python
n = 3
list1 = [1, 2, 3, 4, 5]
list2 = ['a', 'b', 'c', 'd', 'e']
list1[n:], list2[n:] = list2[n:], list1[n:] # 交换第n位后的元素
result = list1 + list2 # 合并两个列表
print(result)
```
输出结果为:
```
[1, 2, 3, 'd', 'e', 'c', 'b', 'a']
```
其中,`list1[n:]` 和 `list2[n:]` 表示从第n位开始到列表末尾的切片,`list1[n:], list2[n:]` 用于同时对两个切片进行赋值,实现元素交换。最后,通过列表拼接将两个列表合并成一个。
相关问题
python实现feistel加解密过程(包括平衡和非平衡两种),轮函数自己设计,对每一部分代码进行注释
Feistel加密是一种块加密算法,其核心思想是将明文分块,通过多次迭代运算,得到密文。在每次迭代运算中,采用分组密码中常用的轮函数对明文进行加密,然后将加密结果与明文的一部分异或,得到下一轮的明文。
Feistel加密可以分为平衡Feistel和非平衡Feistel两种。平衡Feistel指每次迭代中将明文分为两个相等的部分,而非平衡Feistel则将明文分为两个不相等的部分。下面我们将分别实现这两种Feistel加密算法。
## 平衡Feistel加密
平衡Feistel加密的轮函数通常由两个步骤组成:置换和代换。置换过程通过将明文分为两个相等的部分,并将一部分与另一部分进行置换,从而达到混淆的目的。代换过程则通过采用代换表或S盒等方式,对置换后的结果进行替换,进一步增加密文的随机性。
下面是一个简单的平衡Feistel加密实现,其中轮函数采用了置换和代换两个步骤:
``` python
import random
# 将明文分为两个相等的部分
def split_half(text):
n = len(text)
return text[:n//2], text[n//2:]
# 将一部分与另一部分进行置换
def permute(text):
n = len(text)
permuted_text = ""
for i in range(n//2):
permuted_text += text[i+n//2] + text[i]
return permuted_text
# 定义代换表
substitution_table = {
"0000": "1111",
"0001": "1100",
"0010": "1010",
"0011": "1001",
"0100": "0110",
"0101": "0101",
"0110": "0011",
"0111": "0000",
"1000": "1011",
"1001": "1000",
"1010": "0111",
"1011": "0100",
"1100": "0010",
"1101": "0001",
"1110": "1110",
"1111": "1101"
}
# 代换函数
def substitute(text):
substituted_text = ""
for i in range(0, len(text), 4):
block = text[i:i+4]
substituted_block = substitution_table[block]
substituted_text += substituted_block
return substituted_text
# 定义Feistel轮函数
def round_function(text, key):
permuted_text = permute(text)
substituted_text = substitute(permuted_text)
xor_result = int(substituted_text, 2) ^ int(key, 2)
return '{:08b}'.format(xor_result)
# 加密函数
def encrypt(plaintext, key, num_rounds):
left, right = split_half(plaintext)
for i in range(num_rounds):
new_right = int(left, 2) ^ int(round_function(right, key), 2)
left = right
right = '{:08b}'.format(new_right)
ciphertext = right + left
return ciphertext
# 解密函数
def decrypt(ciphertext, key, num_rounds):
left, right = split_half(ciphertext)
for i in range(num_rounds):
new_left = int(right, 2) ^ int(round_function(left, key), 2)
right = left
left = '{:08b}'.format(new_left)
plaintext = left + right
return plaintext
# 测试加密和解密函数
plaintext = "01011010"
key = "10101010"
num_rounds = 3
ciphertext = encrypt(plaintext, key, num_rounds)
decrypted_plaintext = decrypt(ciphertext, key, num_rounds)
print("Plaintext: ", plaintext)
print("Ciphertext: ", ciphertext)
print("Decrypted text: ", decrypted_plaintext)
```
在这个实现中,我们通过 `split_half` 函数将明文分为两个相等的部分,然后将一部分与另一部分进行置换。其中,置换过程中我们使用了Python中的切片操作,从而可以快速地将字符串进行拼接。接着,我们使用了一个代换表,将置换后的结果替换成新的结果。最后,将代换后的结果与密钥进行异或操作,得到本轮的加密结果。
在加密和解密函数中,我们采用了类似于分组密码的结构,将明文和密文分别分为两个部分,并在每轮迭代中交换这两个部分。注意,在进行加密和解密时,使用的轮函数和密钥必须完全一致。
## 非平衡Feistel加密
非平衡Feistel加密与平衡Feistel加密的主要区别在于,明文被分为两个不相等的部分。通常情况下,非平衡Feistel加密的轮函数采用了更加复杂的设计,以达到更好的加密效果。
下面是一个简单的非平衡Feistel加密实现,其中轮函数采用了置换和代换两个步骤:
``` python
import random
# 将明文分为两个不相等的部分
def split(text):
n = len(text)
split_index = random.randint(1, n-1)
return text[:split_index], text[split_index:]
# 将一部分与另一部分进行置换
def permute(text):
n = len(text)
permuted_text = ""
for i in range(n//2):
permuted_text += text[i+n//2] + text[i]
return permuted_text
# 定义代换表
substitution_table = {
"0000": "1111",
"0001": "1100",
"0010": "1010",
"0011": "1001",
"0100": "0110",
"0101": "0101",
"0110": "0011",
"0111": "0000",
"1000": "1011",
"1001": "1000",
"1010": "0111",
"1011": "0100",
"1100": "0010",
"1101": "0001",
"1110": "1110",
"1111": "1101"
}
# 代换函数
def substitute(text):
substituted_text = ""
for i in range(0, len(text), 4):
block = text[i:i+4]
substituted_block = substitution_table[block]
substituted_text += substituted_block
return substituted_text
# 定义Feistel轮函数
def round_function(text, key):
permuted_text = permute(text)
substituted_text = substitute(permuted_text)
xor_result = int(substituted_text, 2) ^ int(key, 2)
return '{:08b}'.format(xor_result)
# 加密函数
def encrypt(plaintext, key, num_rounds):
left, right = split(plaintext)
for i in range(num_rounds):
new_right = int(left, 2) ^ int(round_function(right, key), 2)
left = right
right = '{:08b}'.format(new_right)
ciphertext = right + left
return ciphertext
# 解密函数
def decrypt(ciphertext, key, num_rounds):
left, right = split(ciphertext)
for i in range(num_rounds):
new_left = int(right, 2) ^ int(round_function(left, key), 2)
right = left
left = '{:08b}'.format(new_left)
plaintext = left + right
return plaintext
# 测试加密和解密函数
plaintext = "01011010"
key = "10101010"
num_rounds = 3
ciphertext = encrypt(plaintext, key, num_rounds)
decrypted_plaintext = decrypt(ciphertext, key, num_rounds)
print("Plaintext: ", plaintext)
print("Ciphertext: ", ciphertext)
print("Decrypted text: ", decrypted_plaintext)
```
在这个实现中,我们采用了 `random` 模块生成随机数,将明文分为两个不相等的部分,并将一部分与另一部分进行置换。代换函数和Feistel轮函数的实现与平衡Feistel加密相同,这里不再赘述。
需要注意的是,在非平衡Feistel加密中,每轮迭代中的轮函数设计非常重要。通常情况下,我们需要使用更加复杂的轮函数,以达到更好的加密效果。
创建一个带头结点的双链表,链表中元素随机排列,逆置该链表
好的,为您解答。
以下是创建带头结点的双链表,链表中元素随机排列,并逆置该链表的代码,供您参考。
```python
# 定义双向链表节点类
class Node(object):
def __init__(self, val=None):
self.val = val
self.next = None
self.prev = None
# 定义双向链表类
class DoubleLinkedList(object):
def __init__(self):
self.head = Node()
self.tail = Node()
self.head.next = self.tail
self.tail.prev = self.head
# 在链表尾部插入节点
def add_node(self, val):
node = Node(val)
node.next = self.tail
node.prev = self.tail.prev
self.tail.prev.next = node
self.tail.prev = node
# 随机生成链表元素并插入
def create_random_list(self, n):
import random
for i in range(n):
val = random.randint(1, 100)
self.add_node(val)
# 反转链表
def reverse_list(self):
cur = self.head.next
while cur != self.tail:
cur.next, cur.prev = cur.prev, cur.next
cur = cur.prev
self.head.next, self.tail.prev = self.tail.prev, self.head.next
self.head.next.prev = self.head
self.tail.prev.next = self.tail
# 打印链表所有节点的值
def print_list(self):
cur = self.head.next
while cur != self.tail:
print(cur.val, end=' ')
cur = cur.next
# 在实际使用时,创建一个DoubleLinkedList对象,随机生成元素并插入,然后反转链表
if __name__ == '__main__':
dl = DoubleLinkedList()
dl.create_random_list(10)
print('原始链表:')
dl.print_list()
print('\n反转后的链表:')
dl.reverse_list()
dl.print_list()
```
其中,`create_random_list(n)` 方法可以创建一个 n 个元素的双向随机链表,`reverse_list()` 方法可以将链表反转。具体实现中将反转操作分成两步执行,首先将所有节点的 next 与 prev 交换,反转后 head 和 tail 的 next 与 prev 也需要调换。
相关推荐









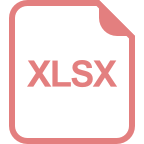
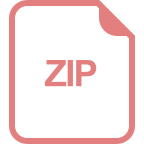