在linux上最精确的获取时间戳方法
时间: 2024-05-16 12:13:57 浏览: 14
在Linux上最精确的获取时间戳方法是使用`clock_gettime()`函数。该函数使用高精度计时器来获取当前时间。以下是一个使用`clock_gettime()`函数获取时间戳的示例代码:
```
#include <time.h>
int main() {
struct timespec ts;
clock_gettime(CLOCK_MONOTONIC_RAW, &ts);
uint64_t timestamp = ts.tv_sec * 1000000 + ts.tv_nsec / 1000;
printf("Timestamp: %lu\n", timestamp);
return 0;
}
```
在上述示例代码中,使用`CLOCK_MONOTONIC_RAW`参数指定了使用系统的硬件时钟来获取时间戳。该函数返回秒和纳秒级别的时间。在代码中将其转换为微秒级别的时间戳。
相关问题
在linux上最精确的获取时间戳方法,精确到微妙
在 Linux 上,可以使用 `clock_gettime()` 函数获取更高精度的时间戳,精确到纳秒级别。以下是获取时间戳精确到微秒的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
struct timespec ts;
if (clock_gettime(CLOCK_REALTIME, &ts) == -1) {
perror("clock_gettime");
exit(EXIT_FAILURE);
}
printf("Timestamp: %ld.%06ld\n", ts.tv_sec, ts.tv_nsec / 1000);
return 0;
}
```
在上面的代码中,`clock_gettime()` 函数的第一个参数为时钟类型,这里使用 `CLOCK_REALTIME` 表示系统实时时间时钟。第二个参数为存储时间戳的结构体指针。获取到的时间戳中,`tv_sec` 表示秒数,`tv_nsec` 表示纳秒数,将纳秒数除以 1000 即可得到微秒数。
c linux 获取当前时间
在 C 语言中,可以使用 `time()` 函数来获取当前的 Unix 时间戳。时间戳是从 1970 年 1 月 1 日(UTC)开始计算的秒数。
下面是一个例子,展示了如何使用 `time()` 函数获取当前的 Unix 时间戳并将其转换为本地时间:
```
#include <stdio.h>
#include <time.h>
int main(void) {
time_t current_time = time(NULL); // 获取当前的 Unix 时间戳
struct tm *local_time = localtime(¤t_time); // 将时间戳转换为本地时间
printf("当前时间:%d年%d月%d日 %d:%d:%d\n",
local_time->tm_year + 1900, local_time->tm_mon + 1, local_time->tm_mday,
local_time->tm_hour, local_time->tm_min, local_time->tm_sec);
return 0;
}
```
需要注意的是,`time()` 函数返回的时间戳是以秒为单位的。如果需要更精确的时间,可以使用 `clock_gettime()` 函数获取当前的时钟时间。
此外,还有许多其他方法可以获取当前时间,比如使用系统调用 `gettimeofday()` 或者使用 `<chrono>` 头文件中的函数。具体方法取决于你的需求和系统平台。
相关推荐
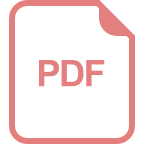
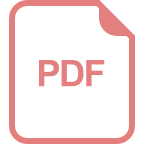
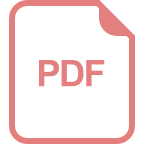
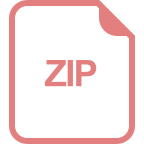
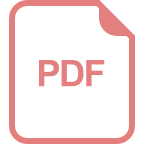
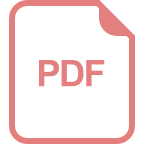
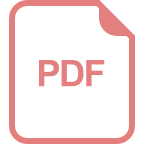
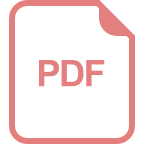
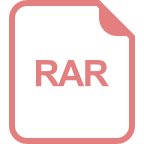
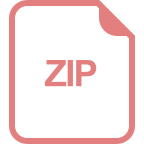
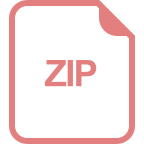
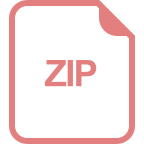
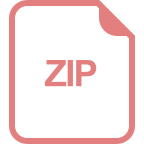
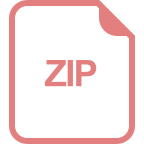