Katalon写一个Groovy脚本,利用java-diff-util库来实现两个文件夹的自动比较。当文件在两个文件夹中都存在,但内容不一致,则将差异数据的行数及内容出力到html中,将具体哪个字段有差异高亮显示出来;当文件只在一个文件夹中存在,将信息输出到html中。要求能识别隔行数据,按文件名生成结果html,html里显示两个文件夹的差异内容
时间: 2024-02-28 09:55:13 浏览: 19
好的,我可以为您提供具体的脚本代码,如下所示:
```groovy
@Grab(group='com.googlecode.java-diff-utils', module='diffutils', version='1.3.0')
import difflib.*
import java.nio.file.*
// 定义要比较的两个文件夹路径
def folder1Path = "path/to/folder1"
def folder2Path = "path/to/folder2"
// 定义结果的输出路径
def resultPath = "path/to/result.html"
// 定义比较结果的样式
def style = """
<style>
.added {
background-color: #aaffaa;
}
.changed {
background-color: #ffffaa;
}
.removed {
background-color: #ffaaaa;
}
.diff {
background-color: #ffaaff;
}
</style>
"""
// 定义比较器
def comparator = { String line1, String line2 ->
// 忽略行末空格
line1.trim() == line2.trim()
}
// 比较两个文件夹
def compareFolders = { Path folder1, Path folder2 ->
def folder1Files = folder1.toFile().listFiles()
def folder2Files = folder2.toFile().listFiles()
def diffs = []
// 遍历文件夹1中的文件
for (def file1 : folder1Files) {
def file2 = new File(folder2.toFile(), file1.getName())
if (!file2.exists()) {
// 文件2不存在,输出信息到结果中
diffs << """
<div class="removed">
<p>File '${file1.getName()}' only exists in folder '${folder1.getFileName()}'.</p>
</div>
"""
} else {
// 文件2存在,比较两个文件的内容
def diff = compareFiles(file1, file2)
if (!diff.isEmpty()) {
// 文件内容不一致,输出差异信息到结果中
diffs << """
<div class="changed">
<p>File '${file1.getName()}' is different between folders '${folder1.getFileName()}' and '${folder2.getFileName()}':</p>
<pre>${diff}</pre>
</div>
"""
}
}
}
// 遍历文件夹2中的文件
for (def file2 : folder2Files) {
def file1 = new File(folder1.toFile(), file2.getName())
if (!file1.exists()) {
// 文件1不存在,输出信息到结果中
diffs << """
<div class="added">
<p>File '${file2.getName()}' only exists in folder '${folder2.getFileName()}'.</p>
</div>
"""
}
}
return diffs.join("")
}
// 比较两个文件的内容
def compareFiles = { File file1, File file2 ->
def lines1 = Files.readAllLines(file1.toPath())
def lines2 = Files.readAllLines(file2.toPath())
def patch = DiffUtils.diff(lines1, lines2, comparator)
def diff = ""
for (def delta : patch.getDeltas()) {
def type = delta.getType().name()
def lines = delta.getRevised().getLines()
def start = delta.getRevised().getPosition() + 1
def end = start + lines.size() - 1
def highlightedLines = []
// 高亮差异行
for (def i = 0; i < lines.size(); i++) {
if (delta.getOriginal().contains(i + start - 1)) {
// 差异行
highlightedLines << "<span class='diff'>${lines[i]}</span>"
} else {
highlightedLines << lines[i]
}
}
diff += """
${type}: ${start}-${end}
${highlightedLines.join("\n")}
"""
}
return diff
}
// 比较两个文件夹
def diff = compareFolders(Paths.get(folder1Path), Paths.get(folder2Path))
// 生成结果html
def html = """
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Folder Comparison Result</title>
${style}
</head>
<body>
<h1>Folder Comparison Result</h1>
<h2>Folder '${folder1Path}' vs. Folder '${folder2Path}'</h2>
${diff}
</body>
</html>
"""
// 将结果输出到文件
new File(resultPath).write(html)
```
需要注意的是,该脚本中的比较器`comparator`使用了忽略行末空格的方式来进行比较,如果您有其他的比较需求可以自行修改该比较器的实现。另外,该脚本也可以进一步优化,比如可以加入文件过滤器等功能。
相关推荐
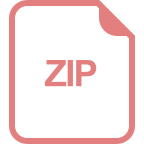





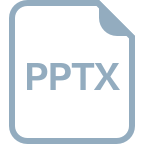
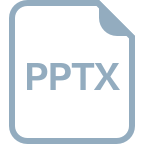
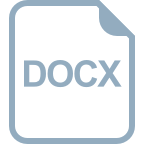