(1)首先,定义字符串:myStr = "星期一星期二星期三星期四星 期五星期六星期日",然后,编写程序通过 input()函数读入一个表 示星期几的数字(1-7),输出对应的星期字符串,例如:输入
时间: 2024-09-20 12:10:17 浏览: 94
当你需要编写一个程序,让用户通过输入一个数字(1到7表示一周中的每一天)并获取对应的星期名称时,可以按照以下步骤操作:
1. 首先,将给定的字符串按照星期分隔存储在一个列表或数组中,就像你提到的那样:
```python
week_days = ["星期一", "星期二", "星期三", "星期四", "星期五", "星期六", "星期日"]
```
2. 然后创建一个字典或映射,将数字作为键,星期名称作为值,方便查找:
```python
days_dict = {1: '星期一', 2: '星期二', ..., 7: '星期日'}
```
3. 使用`input()`函数接收用户的输入,并转换成整数类型:
```python
user_input = int(input("请输入代表星期的数字(1-7):"))
```
4. 根据用户输入,在字典中找到对应的星期名称:
```python
weekday_str = days_dict.get(user_input)
```
5. 最后,输出相应的星期字符串:
```python
if weekday_str is not None:
print(f"今天是{weekday_str}。")
else:
print("无效的输入!")
```
相关问题
要求:下列各题要求打印输出结果。 一,查找 定义字符串: mystr = hello world and itcast and itheima and python 按下列要求对mystr字符串进行操作,并将结果打印输出. 1.find( (1)查找and子串出现位置下标 (2
在Python中,可以使用`find()`函数来查找子串在字符串中的位置。对于给定的字符串`mystr = "hello world and itcast and itheima and python"`,我们可以按照您的要求进行操作:
1. 查找"and"子串出现的位置下标:
```python
mystr = "hello world and itcast and itheima and python"
index = mystr.find("and")
print(f"And子串第一次出现的位置是:{index}")
```
这里注意,`find()`返回的是子串首次出现的位置,如果没有找到则返回-1。
2. 查找"python"子串出现的位置下标:
```python
py_index = mystr.find("python")
print(f"Python子串出现的位置是:{py_index}")
```
同样,如果没找到会返回-1。
3. 如果想查找所有"and"子串出现的位置,可以循环查找并存储:
```python
all_indices = [mystr.find("and", i) for i in range(len(mystr))]
print("所有的'and'子串位置:", all_indices)
```
这将返回一个列表,包含每个"and"子串首次出现的所有索引。
给定一个字符串,如: mystr = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789" 使用所学的知识,从字符串中随机取出4个字符,且验证码必须包含大写字母,小写字母和数字,生成验证码。
好的,您可以使用 Python 中的 random 模块来实现此功能。以下是一段代码可以实现您的需求:
```python
import random
mystr = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"
code = ''
while True:
for i in range(4):
code += random.choice(mystr)
if any(c.isupper() for c in code) and any(c.islower() for c in code) and any(c.isdigit() for c in code):
# 验证码中包含大写字母、小写字母和数字,结束循环
break
else:
code = '' # 重新生成验证码
print(code)
```
程序会不断生成验证码,直到满足条件:包含大写字母、小写字母和数字。生成的验证码会打印出来。希望这个解答可以帮到您!
阅读全文
相关推荐
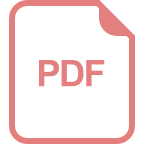
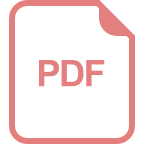
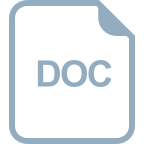













