python中将字典按值排序
时间: 2023-11-08 14:48:48 浏览: 51
在 Python 中,可以使用 `sorted()` 函数以及 `key` 参数来对字典按值排序。下面是一个例子:
```python
my_dict = {'a': 5, 'b': 2, 'c': 3, 'd': 1}
sorted_dict = sorted(my_dict.items(), key=lambda x: x[1])
print(sorted_dict)
```
输出结果将会是:
```
[('d', 1), ('b', 2), ('c', 3), ('a', 5)]
```
这里我们使用 `items()` 方法将字典转换为一个包含键值对的元组列表。然后,通过 `key` 参数指定排序的依据,这里使用 `lambda` 函数来指定按值排序。最后,使用 `sorted()` 函数对这个列表进行排序。
相关问题
python的字典按值排序
以下是Python中按字典值排序的方法:
1.使用sorted()函数和lambda表达式
```python
# 定义一个字典
my_dict = {'apple': 10, 'orange': 20, 'banana': 5}
# 使用sorted()函数和lambda表达式按值排序
sorted_dict = dict(sorted(my_dict.items(), key=lambda x: x[1]))
# 输出排序后的字典
print(sorted_dict)
```
输出:
```
{'banana': 5, 'apple': 10, 'orange': 20}
```
2.使用operator模块的itemgetter()函数
```python
# 导入operator模块
import operator
# 定义一个字典
my_dict = {'apple': 10, 'orange': 20, 'banana': 5}
# 使用operator模块的itemgetter()函数按值排序
sorted_dict = dict(sorted(my_dict.items(), key=operator.itemgetter(1)))
# 输出排序后的字典
print(sorted_dict)
```
输出:
```
{'banana': 5, 'apple': 10, 'orange': 20}
```
python排序字典按值
在Python中,可以使用内置的sorted()函数和lambda函数来对字典按值进行排序。下面是一个示例代码:
```python
# 定义一个字典
my_dict = {'apple': 10, 'banana': 5, 'orange': 8, 'grape': 3}
# 使用sorted()函数和lambda函数按值排序字典
sorted_dict = dict(sorted(my_dict.items(), key=lambda x: x[1]))
# 打印排序后的字典
for key, value in sorted_dict.items():
print(key, value)
```
运行以上代码,输出结果如下:
```
grape 3
banana 5
orange 8
apple 10
```
在这个示例中,我们使用sorted()函数对字典的items()进行排序,key参数指定了排序的依据,这里使用lambda函数来指定按值进行排序。最后,我们将排序后的结果转换为字典类型,并打印出每个键值对。
相关推荐
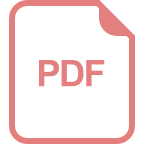
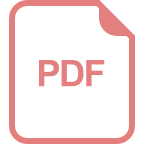
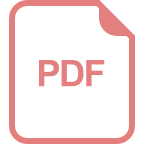












