用Python以及tkinter包完成一个类似于Windows计算器的程序,加分项 程序员计算器功能,科学计算器功能
时间: 2024-03-24 22:39:10 浏览: 135
好的,我可以为您提供一些基本的代码框架来实现这个任务。首先,我们需要导入所需的模块和库,包括tkinter、math等。
```python
from tkinter import *
import math
```
然后,我们需要创建一个名为Calculator的类,该类将包含所有计算器的基本功能。我们还需要定义一些全局变量,例如当前操作数和结果等。
```python
class Calculator:
def __init__(self, master):
self.master = master
self.master.title("Calculator")
self.result = 0
self.current = ""
self.operation = ""
self.display = Entry(master, width=25, font=('Arial', 16))
self.display.grid(row=0, column=0, columnspan=4, padx=5, pady=5)
```
接下来,我们将定义一些函数来处理计算器的不同操作,例如数字输入、运算符输入、清除操作以及计算结果等。
```python
def button_click(self, number):
self.current = str(self.current) + str(number)
self.display.delete(0, END)
self.display.insert(0, self.current)
def button_clear(self):
self.result = 0
self.current = ""
self.operation = ""
self.display.delete(0, END)
def button_add(self):
self.result += float(self.current)
self.current = ""
self.operation = "+"
self.display.delete(0, END)
def button_subtract(self):
self.result -= float(self.current)
self.current = ""
self.operation = "-"
self.display.delete(0, END)
def button_multiply(self):
self.result *= float(self.current)
self.current = ""
self.operation = "*"
self.display.delete(0, END)
def button_divide(self):
self.result /= float(self.current)
self.current = ""
self.operation = "/"
self.display.delete(0, END)
def button_equal(self):
if self.operation == "+":
self.result += float(self.current)
elif self.operation == "-":
self.result -= float(self.current)
elif self.operation == "*":
self.result *= float(self.current)
elif self.operation == "/":
self.result /= float(self.current)
self.display.delete(0, END)
self.display.insert(0, self.result)
self.result = 0
self.current = ""
self.operation = ""
```
最后,我们将添加所有必要的按钮,并将它们绑定到相应的函数。
```python
root = Tk()
calc = Calculator(root)
button_1 = Button(root, text="1", padx=20, pady=10, command=lambda: calc.button_click(1))
button_2 = Button(root, text="2", padx=20, pady=10, command=lambda: calc.button_click(2))
button_3 = Button(root, text="3", padx=20, pady=10, command=lambda: calc.button_click(3))
button_4 = Button(root, text="4", padx=20, pady=10, command=lambda: calc.button_click(4))
button_5 = Button(root, text="5", padx=20, pady=10, command=lambda: calc.button_click(5))
button_6 = Button(root, text="6", padx=20, pady=10, command=lambda: calc.button_click(6))
button_7 = Button(root, text="7", padx=20, pady=10, command=lambda: calc.button_click(7))
button_8 = Button(root, text="8", padx=20, pady=10, command=lambda: calc.button_click(8))
button_9 = Button(root, text="9", padx=20, pady=10, command=lambda: calc.button_click(9))
button_0 = Button(root, text="0", padx=20, pady=10, command=lambda: calc.button_click(0))
button_add = Button(root, text="+", padx=19, pady=10, command=calc.button_add)
button_subtract = Button(root, text="-", padx=20, pady=10, command=calc.button_subtract)
button_multiply = Button(root, text="*", padx=20, pady=10, command=calc.button_multiply)
button_divide = Button(root, text="/", padx=20, pady=10, command=calc.button_divide)
button_clear = Button(root, text="C", padx=19, pady=10, command=calc.button_clear)
button_equal = Button(root, text="=", padx=20, pady=10, command=calc.button_equal)
button_1.grid(row=1, column=0)
button_2.grid(row=1, column=1)
button_3.grid(row=1, column=2)
button_add.grid(row=1, column=3)
button_4.grid(row=2, column=0)
button_5.grid(row=2, column=1)
button_6.grid(row=2, column=2)
button_subtract.grid(row=2, column=3)
button_7.grid(row=3, column=0)
button_8.grid(row=3, column=1)
button_9.grid(row=3, column=2)
button_multiply.grid(row=3, column=3)
button_clear.grid(row=4, column=0)
button_0.grid(row=4, column=1)
button_equal.grid(row=4, column=2)
button_divide.grid(row=4, column=3)
root.mainloop()
```
这是一个基本的计算器,您可以在此基础上添加更多的功能,例如科学计算器功能、程序员计算器功能等。
阅读全文
相关推荐




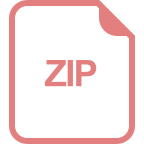
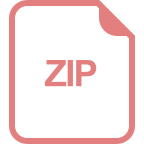
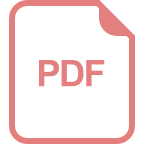
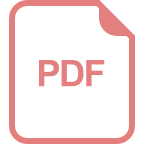
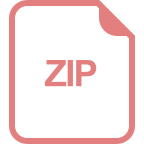
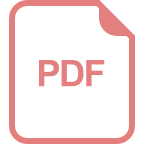
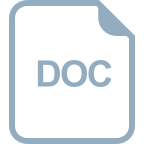
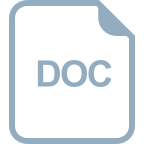
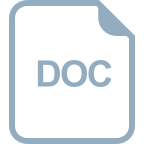


