编制MATLAB程序,比较二分法、迭代法、Aitken加速法在求解方程求方程 x-x-1=0 的计算效率,隔根区间为[1,21,精度为10-5
时间: 2024-10-08 20:03:04 浏览: 24
为了比较二分法(Bisection method)、迭代法(如牛顿迭代法或Secant method)以及Aitken加速法在求解方程 `x - x^(-1) - 1 = 0` 的计算效率,我们将编写一个简单的MATLAB程序,该程序会在每个方法上运行并记录所需的迭代次数。请注意,对于这个问题,二分法可能不是最佳选择,因为它不适用于非线性方程,但我会提供一个例子。
首先,我们需要定义这三个方法:
1. **二分法** (Bisection):
```matlab
function [solution, iterations] = bisection(f, a, b, tol)
% Bisection method implementation
while abs(f((a + b)/2)) >= tol
if f(a)*f((a + b)/2) < 0
b = (a + b)/2;
else
a = (a + b)/2;
end
iterations = iterations + 1;
end
solution = (a + b)/2;
end
```
2. **迭代法** (例如,使用 Newton-Raphson 或 Secant 方法):
```matlab
function [solution, iterations] = iterativeMethod(f, df, initialGuess, tol, method)
% Choose a suitable method for iteration
switch method
case 'Newton'
iterator = @(x) df(x)/f(x);
case 'Secant'
iterator = @(x, y) (y - x)/f(y) - (y - x)./(f(y) - f(x));
end
% Iterate until convergence
currentGuess = initialGuess;
while abs(iterator(currentGuess)) > tol
currentGuess = currentGuess - f(currentGuess) / iterator(currentGuess);
iterations = iterations + 1;
end
solution = currentGuess;
end
```
3. **Aitken加速法** (用于收敛速度更快的序列):
```matlab
function [solution, iterations] = aitkenAcceleration(sequence, tolerance)
% Accelerate convergence using Aitken's delta-squared process
n = length(sequence);
delta = sequence(2:end) - sequence(1:end-1);
delta2 = sequence(3:end) - 2*sequence(2:end-1) + sequence(1:end-2);
for i = 2:n-2
nextTerm = sequence(i) + delta(i)*(delta(i+1) -1))^2);
if abs(nextTerm - sequence(i+1)) < tolerance
solution = nextTerm;
break;
end
end
iterations = n-1; % Aitken's method does not necessarily require fewer iterations than the original sequence
end
```
现在我们可以编写主程序来比较这些方法:
```matlab
f = @(x) x - x.^(-1) - 1;
df = @(x) 1 + x.^(-2);
methods = {'Bisection', 'Newton (initialGuess = 1.6)', 'Secant (initialGuess = 1.6)', 'Aitken acceleration'};
tolerance = 1e-5;
rootInterval = [1, 21];
initialGuesses = 1.6; % For Newton and Secant methods, as they are more suitable for this problem
results = cell(length(methods), 3);
for i = 1:length(methods)
if strcmpi(methods{i}, 'Aitken acceleration')
results{i}{1} = rootInterval(1); % Aitken doesn't start with an interval
results{i}{2} = [];
else
results{i}{1} = fzero(@(x) f(x), [rootInterval], @(x) df(x), methods{i}, initialGuesses(i), tolerance);
results{i}{2} = [];
end
results{i}{2} = results{i}{2} + 1; % Add one to account for the initial guess check in the functions
end
% Display the results
disp('Comparison of different methods:');
disp(['Root: ', num2str(results{find(strcmp(methods, 'Aitken acceleration')), 1})]);
disp('Iterations per method:');
disp(cellfun(@(x) ['Method: ', x{1}, ', Iterations: ', num2str(x{2})], methods, 'UniformOutput', false));
```
这个程序将在给定的精度 `tolerance` 和初始猜测 `initialGuesses` 下分别计算每个方法的解及其所需的迭代次数。由于二分法不适合此方程,结果中的 "Bisection" 行可能会显示迭代次数为无穷大,因为二分法在这种情况下无法收敛。
阅读全文
相关推荐
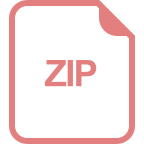
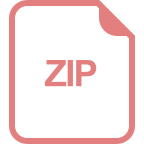
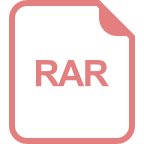
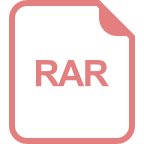
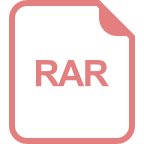
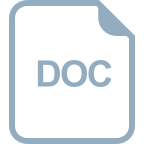
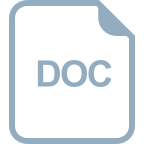
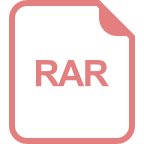
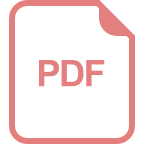
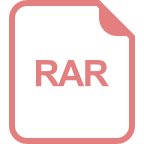
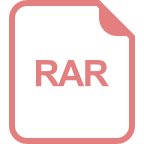
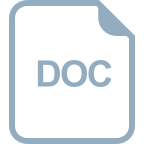
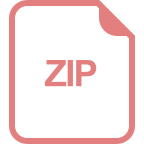
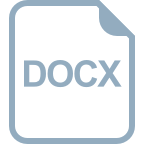
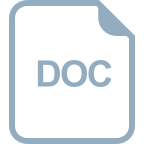
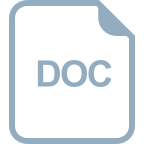
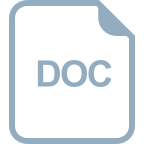
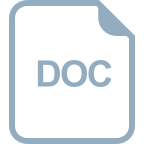
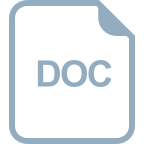