使用jquery完成九九乘法表、全选/全不选、反选功能、轮播图
时间: 2023-09-17 16:11:13 浏览: 92
以下是使用 jQuery 实现九九乘法表、全选/全不选、反选功能和轮播图的示例代码:
1. 九九乘法表:
```html
<!DOCTYPE html>
<html>
<head>
<title>九九乘法表</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<table id="multiplication-table">
<tbody></tbody>
</table>
<script>
$(document).ready(function() {
var table = $('#multiplication-table tbody');
for (var i = 1; i <= 9; i++) {
var row = $('<tr></tr>');
for (var j = 1; j <= 9; j++) {
var cell = $('<td></td>').text(i + ' × ' + j + ' = ' + (i * j));
row.append(cell);
}
table.append(row);
}
});
</script>
</body>
</html>
```
2. 全选/全不选、反选功能:
```html
<!DOCTYPE html>
<html>
<head>
<title>全选/全不选、反选功能</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<label><input type="checkbox" id="check-all"> 全选/全不选</label><br>
<input type="checkbox" class="checkbox-item"> Item 1<br>
<input type="checkbox" class="checkbox-item"> Item 2<br>
<input type="checkbox" class="checkbox-item"> Item 3<br>
<button id="invert-selection">反选</button>
<script>
$(document).ready(function() {
$('#check-all').change(function() {
$('.checkbox-item').prop('checked', $(this).prop('checked'));
});
$('#invert-selection').click(function() {
$('.checkbox-item').each(function() {
$(this).prop('checked', !$(this).prop('checked'));
});
});
});
</script>
</body>
</html>
```
3. 轮播图:
```html
<!DOCTYPE html>
<html>
<head>
<title>轮播图</title>
<style>
.slider {
width: 300px;
height: 200px;
overflow: hidden;
position: relative;
}
.slider img {
width: 100%;
height: 100%;
position: absolute;
transition: opacity 1s ease-in-out;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="slider">
<img src="image1.jpg" alt="Image 1">
<img src="image2.jpg" alt="Image 2">
<img src="image3.jpg" alt="Image 3">
</div>
<script>
$(document).ready(function() {
var slider = $('.slider');
var images = slider.find('img');
var currentIndex = 0;
function showImage(index) {
images.css('opacity', 0);
$(images[index]).css('opacity', 1);
}
function nextImage() {
currentIndex = (currentIndex + 1) % images.length;
showImage(currentIndex);
}
setInterval(nextImage, 2000);
});
</script>
</body>
</html>
```
请注意替换示例中的图片路径和文件名,确保图片可以正确加载。希望能对你有所帮助!
阅读全文
相关推荐
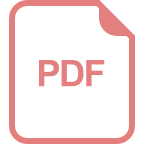
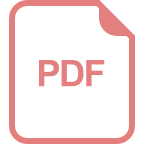
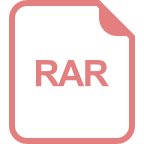
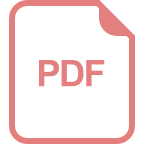
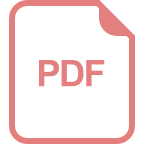
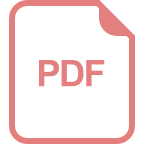
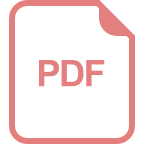
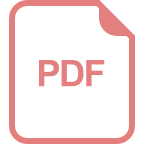
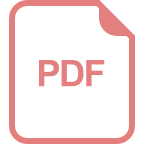
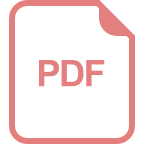
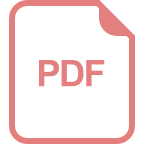
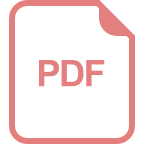
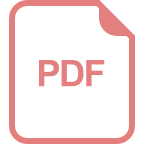
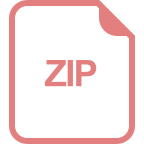
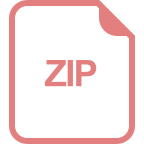
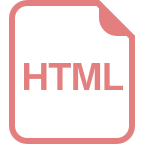