C语言使用可变参数函数编写对动态链表某一节点的查找
时间: 2023-06-04 11:08:09 浏览: 94
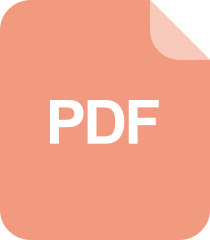
C语言中编写可变参数函数

好的,我可以回答这个问题。您可以使用可变参数函数 va_list、va_start、va_arg 和 va_end 来完成此任务。具体代码实现如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <stdarg.h>
struct node {
int data;
struct node *next;
};
struct node *search_node(int n, ...) {
va_list args;
struct node *cur_node;
int i;
va_start(args, n);
cur_node = va_arg(args, struct node *);
for (i = 1; i < n; i++) {
cur_node = cur_node->next;
}
va_end(args);
return cur_node;
}
int main() {
struct node *head, *cur_node;
int i;
/* Initialize dynamic linked list */
head = malloc(sizeof(struct node));
head->data = 0;
head->next = NULL;
cur_node = head;
for (i = 1; i < 10; i++) {
cur_node->next = malloc(sizeof(struct node));
cur_node = cur_node->next;
cur_node->data = i;
cur_node->next = NULL;
}
/* Search for node */
cur_node = search_node(5, head, head->next->next, head->next->next->next->next, head->next->next->next->next->next->next);
printf("Found node with data = %d\n", cur_node->data);
return 0;
}
```
阅读全文
相关推荐
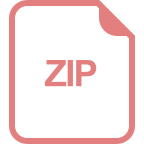
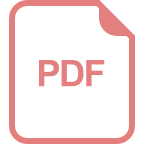
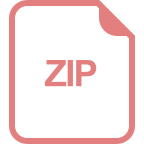
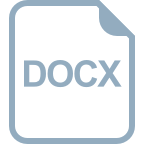
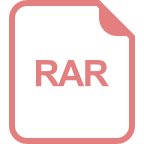
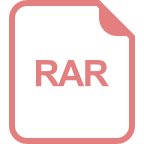
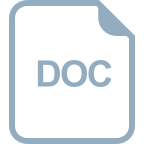
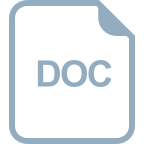
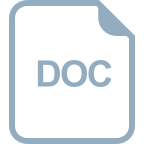
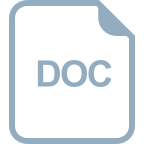
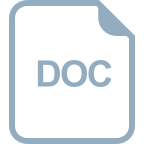
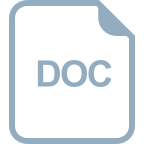
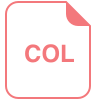
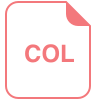
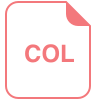
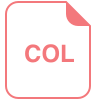
