基于python和opencv、mysql的车牌识别系统
时间: 2023-09-06 11:06:48 浏览: 55
好的,这是一个基于Python和OpenCV、MySQL的车牌识别系统的简单示例代码。请注意,这只是一个简单的示例代码,实际实现可能需要更多的功能和细节。
```python
import cv2
import numpy as np
import pytesseract
import mysql.connector
# 创建MySQL连接
mydb = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="database"
)
# 加载图像
img = cv2.imread('car.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 进行边缘检测
edges = cv2.Canny(gray, 100, 200)
# 进行形态学操作
kernel = np.ones((3, 3), np.uint8)
closing = cv2.morphologyEx(edges, cv2.MORPH_CLOSE, kernel)
# 查找轮廓
contours, hierarchy = cv2.findContours(closing, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 筛选车牌轮廓
for cnt in contours:
area = cv2.contourArea(cnt)
if area > 5000:
x, y, w, h = cv2.boundingRect(cnt)
aspect_ratio = float(w) / h
if aspect_ratio > 2.5 and aspect_ratio < 5:
# 提取车牌区域图像
plate_img = gray[y:y+h, x:x+w]
# 识别车牌号码
plate_number = pytesseract.image_to_string(plate_img, config='--psm 11')
# 插入MySQL数据库
mycursor = mydb.cursor()
sql = "INSERT INTO plates (number, image) VALUES (%s, %s)"
val = (plate_number, cv2.imencode('.jpg', plate_img)[1].tobytes())
mycursor.execute(sql, val)
mydb.commit()
# 显示结果
cv2.imshow('plate detection', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,这个示例代码中,使用了pytesseract库来进行车牌号码的识别,并将识别结果和车牌图像一起存储到MySQL数据库中。同时,你需要创建一个名为“plates”的MySQL表格,用于存储车牌号码和图像。
相关推荐
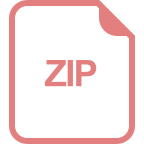
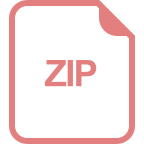
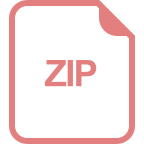














