帮我写一个这种数据转化的代码
时间: 2023-08-20 20:16:09 浏览: 40
好的,以下是一个简单的数据转换代码,用于将 glTF 模型中的 `(position, normal, tangent, texcoord)` 顶点数据转换为 DirectX 12 中的 `(position, normal, tangent, texcoord)` 输入布局:
```cpp
// 定义顶点结构体
struct Vertex {
DirectX::XMFLOAT3 position;
DirectX::XMFLOAT3 normal;
DirectX::XMFLOAT3 tangent;
DirectX::XMFLOAT2 texcoord;
};
// 读取 glTF 中的四个 bufferView,将数据转换为 Vertex 结构体的形式
std::vector<Vertex> vertices;
for (size_t i = 0; i < num_vertices; ++i) {
Vertex vertex;
vertex.position = DirectX::XMFLOAT3(position_ptr[i * 3], position_ptr[i * 3 + 1], position_ptr[i * 3 + 2]);
vertex.normal = DirectX::XMFLOAT3(normal_ptr[i * 3], normal_ptr[i * 3 + 1], normal_ptr[i * 3 + 2]);
vertex.tangent = DirectX::XMFLOAT3(tangent_ptr[i * 3], tangent_ptr[i * 3 + 1], tangent_ptr[i * 3 + 2]);
vertex.texcoord = DirectX::XMFLOAT2(texcoord_ptr[i * 2], texcoord_ptr[i * 2 + 1]);
vertices.push_back(vertex);
}
// 创建顶点缓冲区
ComPtr<ID3D12Resource> vertexBuffer;
D3D12_HEAP_PROPERTIES heapProps = CD3DX12_HEAP_PROPERTIES(D3D12_HEAP_TYPE_DEFAULT);
D3D12_RESOURCE_DESC bufferDesc = CD3DX12_RESOURCE_DESC::Buffer(vertices.size() * sizeof(Vertex));
ThrowIfFailed(device->CreateCommittedResource(&heapProps, D3D12_HEAP_FLAG_NONE, &bufferDesc,
D3D12_RESOURCE_STATE_COPY_DEST, nullptr, IID_PPV_ARGS(&vertexBuffer)));
// 将顶点数据写入到顶点缓冲区中
UINT8* mappedData = nullptr;
ThrowIfFailed(vertexBuffer->Map(0, nullptr, reinterpret_cast<void**>(&mappedData)));
memcpy(mappedData, vertices.data(), vertices.size() * sizeof(Vertex));
vertexBuffer->Unmap(0, nullptr);
// 定义输入布局
const D3D12_INPUT_ELEMENT_DESC inputLayout[] = {
{ "POSITION", 0, DXGI_FORMAT_R32G32B32_FLOAT, 0, offsetof(Vertex, position), D3D12_INPUT_CLASSIFICATION_PER_VERTEX_DATA, 0 },
{ "NORMAL", 0, DXGI_FORMAT_R32G32B32_FLOAT, 0, offsetof(Vertex, normal), D3D12_INPUT_CLASSIFICATION_PER_VERTEX_DATA, 0 },
{ "TANGENT", 0, DXGI_FORMAT_R32G32B32_FLOAT, 0, offsetof(Vertex, tangent), D3D12_INPUT_CLASSIFICATION_PER_VERTEX_DATA, 0 },
{ "TEXCOORD", 0, DXGI_FORMAT_R32G32_FLOAT, 0, offsetof(Vertex, texcoord), D3D12_INPUT_CLASSIFICATION_PER_VERTEX_DATA, 0 }
};
// 创建输入布局
ComPtr<ID3D12InputLayout> inputLayoutObj;
ThrowIfFailed(device->CreateInputLayout(inputLayout, _countof(inputLayout), vertexShader->GetBufferPointer(),
vertexShader->GetBufferSize(), &inputLayoutObj));
// 绑定顶点缓冲区和输入布局
D3D12_VERTEX_BUFFER_VIEW vertexBufferView = {};
vertexBufferView.BufferLocation = vertexBuffer->GetGPUVirtualAddress();
vertexBufferView.StrideInBytes = sizeof(Vertex);
vertexBufferView.SizeInBytes = vertices.size() * sizeof(Vertex);
commandList->IASetVertexBuffers(0, 1, &vertexBufferView);
commandList->IASetInputLayout(inputLayoutObj.Get());
```
这段代码中,首先将 glTF 中的 `(position, normal, tangent, texcoord)` 顶点数据读取出来,然后将它们按照 `Vertex` 结构体的顺序重新排列,并写入到新的顶点缓冲区中。接着,定义了输入布局的描述信息,并通过 `CreateInputLayout` 函数创建输入布局对象。最后,将顶点缓冲区和输入布局绑定到渲染管线上。
相关推荐
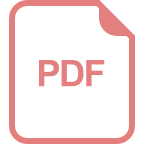
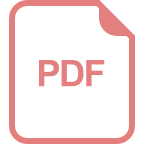
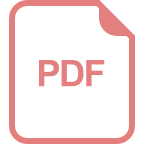



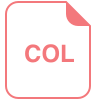










