java设计一个图书信息管理系统,使用Java面向对象技术,使用main主函数,使用异常处理机制,使用jdbc连接my sql数据库
时间: 2023-07-15 16:15:00 浏览: 126
好的,下面是一个简单的图书信息管理系统的设计:
1. 创建一个Book类来存储图书信息,包括书名、作者、出版社、ISBN号码等属性。
```java
public class Book {
private String title;
private String author;
private String publisher;
private String isbn;
public Book(String title, String author, String publisher, String isbn) {
this.title = title;
this.author = author;
this.publisher = publisher;
this.isbn = isbn;
}
// getter and setter methods
}
```
2. 创建一个BookManager类来管理图书信息,包括添加、修改、删除和查询等操作。在这个类中,我们将使用JDBC连接MySQL数据库来实现数据的持久化。
```java
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class BookManager {
private Connection conn;
public BookManager() throws SQLException {
String url = "jdbc:mysql://localhost:3306/book_db"; // 数据库地址
String user = "root"; // 数据库用户名
String password = "123456"; // 数据库密码
conn = DriverManager.getConnection(url, user, password);
}
// 添加图书
public void addBook(Book book) throws SQLException {
String sql = "INSERT INTO books (title, author, publisher, isbn) VALUES (?, ?, ?, ?)";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, book.getTitle());
pstmt.setString(2, book.getAuthor());
pstmt.setString(3, book.getPublisher());
pstmt.setString(4, book.getIsbn());
pstmt.executeUpdate();
}
// 修改图书
public void updateBook(Book book) throws SQLException {
String sql = "UPDATE books SET title=?, author=?, publisher=? WHERE isbn=?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, book.getTitle());
pstmt.setString(2, book.getAuthor());
pstmt.setString(3, book.getPublisher());
pstmt.setString(4, book.getIsbn());
pstmt.executeUpdate();
}
// 删除图书
public void deleteBook(String isbn) throws SQLException {
String sql = "DELETE FROM books WHERE isbn=?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, isbn);
pstmt.executeUpdate();
}
// 查询所有图书
public List<Book> getAllBooks() throws SQLException {
List<Book> books = new ArrayList<>();
String sql = "SELECT * FROM books";
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(sql);
while (rs.next()) {
String title = rs.getString("title");
String author = rs.getString("author");
String publisher = rs.getString("publisher");
String isbn = rs.getString("isbn");
Book book = new Book(title, author, publisher, isbn);
books.add(book);
}
return books;
}
// 根据ISBN号码查询图书
public Book getBookByIsbn(String isbn) throws SQLException {
String sql = "SELECT * FROM books WHERE isbn=?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, isbn);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
String title = rs.getString("title");
String author = rs.getString("author");
String publisher = rs.getString("publisher");
Book book = new Book(title, author, publisher, isbn);
return book;
} else {
return null;
}
}
// 关闭数据库连接
public void close() throws SQLException {
conn.close();
}
}
```
3. 在主函数中,我们可以使用控制台来实现对图书信息的操作,包括添加、修改、删除和查询等。
```java
import java.sql.SQLException;
import java.util.List;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
BookManager manager;
try {
manager = new BookManager();
} catch (SQLException e) {
System.out.println("Failed to connect to database!");
return;
}
while (true) {
System.out.println("Please select an operation:\n1. Add book\n2. Update book\n3. Delete book\n4. List all books\n5. Search book by ISBN\n6. Exit");
int option = scanner.nextInt();
scanner.nextLine();
switch (option) {
case 1:
System.out.println("Please enter the book information (title, author, publisher, ISBN):");
String[] bookInfo = scanner.nextLine().split(",");
if (bookInfo.length != 4) {
System.out.println("Invalid input!");
continue;
}
Book bookToAdd = new Book(bookInfo[0], bookInfo[1], bookInfo[2], bookInfo[3]);
try {
manager.addBook(bookToAdd);
System.out.println("Book added successfully!");
} catch (SQLException e) {
System.out.println("Failed to add book!");
e.printStackTrace();
}
break;
case 2:
System.out.println("Please enter the ISBN of the book to update:");
String isbnToUpdate = scanner.nextLine();
Book bookToUpdate;
try {
bookToUpdate = manager.getBookByIsbn(isbnToUpdate);
} catch (SQLException e) {
System.out.println("Failed to get book information!");
e.printStackTrace();
continue;
}
if (bookToUpdate == null) {
System.out.println("Book not found!");
continue;
}
System.out.println("Please enter the updated book information (title, author, publisher):");
String[] bookInfoToUpdate = scanner.nextLine().split(",");
if (bookInfoToUpdate.length != 3) {
System.out.println("Invalid input!");
continue;
}
bookToUpdate.setTitle(bookInfoToUpdate[0]);
bookToUpdate.setAuthor(bookInfoToUpdate[1]);
bookToUpdate.setPublisher(bookInfoToUpdate[2]);
try {
manager.updateBook(bookToUpdate);
System.out.println("Book updated successfully!");
} catch (SQLException e) {
System.out.println("Failed to update book!");
e.printStackTrace();
}
break;
case 3:
System.out.println("Please enter the ISBN of the book to delete:");
String isbnToDelete = scanner.nextLine();
try {
manager.deleteBook(isbnToDelete);
System.out.println("Book deleted successfully!");
} catch (SQLException e) {
System.out.println("Failed to delete book!");
e.printStackTrace();
}
break;
case 4:
List<Book> books;
try {
books = manager.getAllBooks();
} catch (SQLException e) {
System.out.println("Failed to get book list!");
e.printStackTrace();
continue;
}
if (books.isEmpty()) {
System.out.println("No books found!");
continue;
}
System.out.println("All books:");
for (Book book : books) {
System.out.printf("%s\t%s\t%s\t%s\n", book.getTitle(), book.getAuthor(), book.getPublisher(), book.getIsbn());
}
break;
case 5:
System.out.println("Please enter the ISBN of the book to search:");
String isbnToSearch = scanner.nextLine();
Book bookToSearch;
try {
bookToSearch = manager.getBookByIsbn(isbnToSearch);
} catch (SQLException e) {
System.out.println("Failed to get book information!");
e.printStackTrace();
continue;
}
if (bookToSearch == null) {
System.out.println("Book not found!");
} else {
System.out.printf("%s\t%s\t%s\t%s\n", bookToSearch.getTitle(), bookToSearch.getAuthor(), bookToSearch.getPublisher(), bookToSearch.getIsbn());
}
break;
case 6:
try {
manager.close();
} catch (SQLException e) {
System.out.println("Failed to close database connection!");
e.printStackTrace();
}
System.out.println("Goodbye!");
return;
default:
System.out.println("Invalid option!");
}
}
}
}
```
在这个程序中,我们使用了异常处理机制来处理可能出现的SQLException异常,以避免程序崩溃。同时,我们也使用了面向对象的思想,将图书信息封装在Book类中,并使用BookManager类来管理图书信息。
需要注意的是,这个程序只是一个简单的示例,实际的图书信息管理系统可能会更加复杂,需要根据具体的需求进行设计和实现。
阅读全文
相关推荐
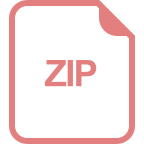
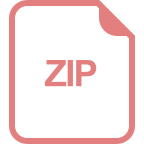
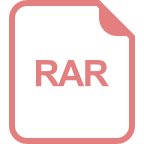
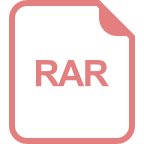
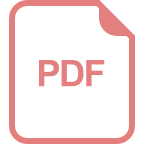
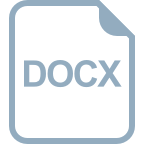
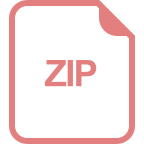
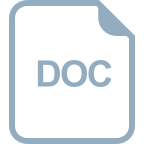
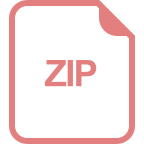
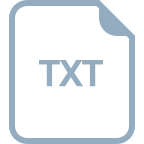
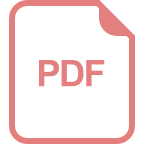
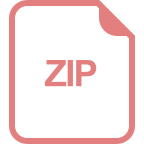
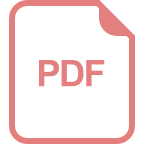
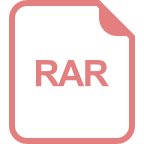
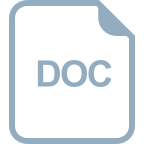
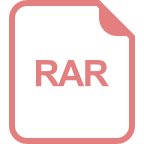
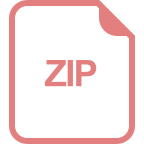
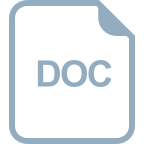