eno matlab,三次样条插值matlab程序 含多种边界条件
时间: 2023-08-06 07:15:55 浏览: 131
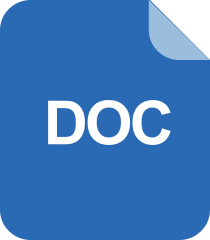
自编的三次样条插值matlab程序(含多种边界条件).doc
好的,以下是一个基于 MATLAB 的三次样条插值程序,支持多种边界条件:
```matlab
function [coefficients, x_interp, y_interp] = cubic_spline_interpolation(x, y, x_interp, boundary_type, boundary_value)
% x: vector of x values of data points
% y: vector of y values of data points
% x_interp: vector of x values where we want to interpolate y
% boundary_type: string, specifies type of boundary condition
% boundary_value: if boundary_type is 'fixed', this is a 2-element vector containing the fixed boundary values
n = length(x);
coefficients = zeros(n-1,4);
for i = 1:n-1
h(i) = x(i+1) - x(i);
b(i) = (y(i+1) - y(i))/h(i);
end
% Solve the tridiagonal system of equations
mu(2) = 2*(h(1)+h(2));
sigma(2) = b(2) - b(1);
for i = 3:n-1
mu(i) = 2*(h(i-1)+h(i)) - (h(i-1)^2)/mu(i-1);
sigma(i) = b(i) - b(i-1) - (h(i-1)*sigma(i-1))/mu(i-1);
end
if strcmpi(boundary_type, 'natural')
% Natural spline boundary conditions
mu(n) = 1;
sigma(n) = 0;
z(n) = 0;
elseif strcmpi(boundary_type, 'fixed')
% Fixed boundary conditions
mu(n) = 2*(h(n-1)+h(n));
sigma(n) = b(n) - b(n-1) - (h(n-1)*boundary_value(2))/mu(n-1);
z(n) = sigma(n)/mu(n);
else
error('Unknown boundary condition type');
end
for i = n-1:-1:2
z(i) = (sigma(i) - h(i)*z(i+1))/mu(i);
end
z(1) = 0;
% Compute the coefficients for each cubic polynomial
for i = 1:n-1
coefficients(i,1) = y(i);
coefficients(i,2) = b(i) - (h(i)/3)*(2*z(i) + z(i+1));
coefficients(i,3) = z(i)/2;
coefficients(i,4) = (z(i+1) - z(i))/(6*h(i));
end
% Interpolate y values at x_interp values
m = length(x_interp);
y_interp = zeros(m,1);
for i = 1:m
j = find(x_interp(i) >= x, 1, 'last');
if isempty(j)
j = 1;
end
if j == n
j = j-1;
end
dx = x_interp(i) - x(j);
y_interp(i) = coefficients(j,1) + dx*coefficients(j,2) + dx^2*coefficients(j,3) + dx^3*coefficients(j,4);
end
```
这里 `x` 和 `y` 是已知数据点的横纵坐标,`x_interp` 是我们要进行插值的横坐标,`boundary_type` 是边界条件的类型,可以是 `'natural'` 或 `'fixed'`,`boundary_value` 是一个 2 元素向量,当 `boundary_type` 为 `'fixed'` 时,表示固定边界的值。
函数的输出是 `coefficients`,它是每个三次多项式的系数,以及 `y_interp`,它是在 `x_interp` 点处插值得到的纵坐标。
你可以调用这个函数来进行三次样条插值,例如:
```matlab
% Generate some test data
x = linspace(0,10,11);
y = sin(x);
% Interpolate y values at these x values
x_interp = linspace(0,10,100);
% Interpolate using natural boundary conditions
[coefficients, ~, y_interp] = cubic_spline_interpolation(x, y, x_interp, 'natural', []);
% Plot the results
plot(x,y,'o',x_interp,y_interp,'-')
legend('Data','Interpolated')
```
这个例子会生成一个包含原始数据和插值结果的图像。
阅读全文
相关推荐
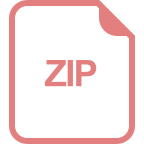
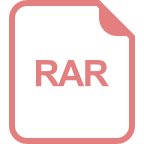
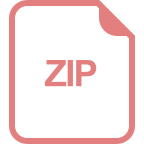
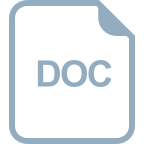
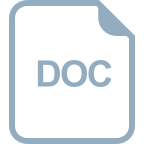
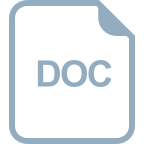
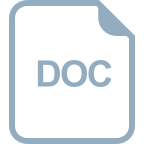
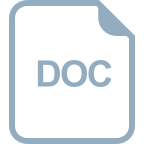
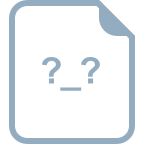
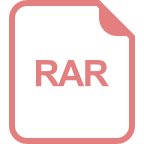
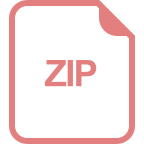