unix domain socket 通信实例
时间: 2023-08-30 08:04:05 浏览: 93
下面是一个简单的 Unix Domain Socket 通信实例,包括了服务端和客户端代码:
服务端代码:
```cpp
#include <sys/socket.h>
#include <sys/un.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define SOCK_PATH "/tmp/echo_socket"
int main(int argc, char *argv[]) {
int s, s2, len;
struct sockaddr_un local, remote;
char str[100];
if ((s = socket(AF_UNIX, SOCK_STREAM, 0)) == -1) {
perror("socket");
exit(1);
}
local.sun_family = AF_UNIX;
strcpy(local.sun_path, SOCK_PATH);
unlink(local.sun_path);
len = strlen(local.sun_path) + sizeof(local.sun_family);
if (bind(s, (struct sockaddr *)&local, len) == -1) {
perror("bind");
exit(1);
}
if (listen(s, 5) == -1) {
perror("listen");
exit(1);
}
printf("Waiting for a connection...\n");
while (1) {
int done, n;
unsigned int t;
t = sizeof(remote);
if ((s2 = accept(s, (struct sockaddr *)&remote, &t)) == -1) {
perror("accept");
exit(1);
}
printf("Connected.\n");
done = 0;
do {
n = recv(s2, str, 100, 0);
if (n <= 0) {
if (n < 0) perror("recv");
done = 1;
}
if (!done) {
if (send(s2, str, n, 0) < 0) {
perror("send");
done = 1;
}
}
} while (!done);
close(s2);
}
return 0;
}
```
客户端代码:
```cpp
#include <sys/socket.h>
#include <sys/un.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define SOCK_PATH "/tmp/echo_socket"
int main(int argc, char *argv[]) {
int s, len;
struct sockaddr_un remote;
char *str = "hello, world!";
if ((s = socket(AF_UNIX, SOCK_STREAM, 0)) == -1) {
perror("socket");
exit(1);
}
remote.sun_family = AF_UNIX;
strcpy(remote.sun_path, SOCK_PATH);
len = strlen(remote.sun_path) + sizeof(remote.sun_family);
if (connect(s, (struct sockaddr *)&remote, len) == -1) {
perror("connect");
exit(1);
}
if (send(s, str, strlen(str), 0) == -1) {
perror("send");
exit(1);
}
if (recv(s, str, strlen(str), 0) == -1) {
perror("recv");
exit(1);
}
printf("Received: %s\n", str);
close(s);
return 0;
}
```
服务端代码创建了一个 Unix Domain Socket 并监听,一旦有客户端连接进来,服务端就接收客户端发送的数据,并将其原样返回。客户端代码连接到服务端,并发送一个字符串,然后等待服务端返回数据并打印。
阅读全文
相关推荐
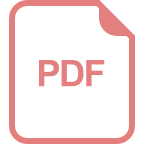
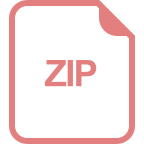
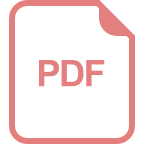
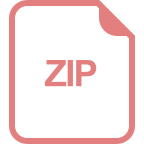
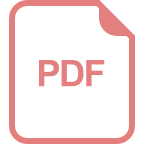
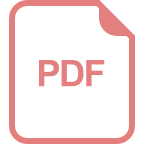
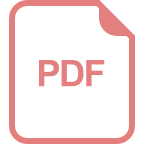
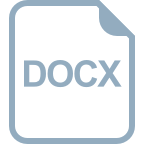
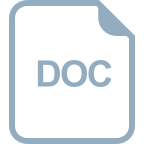
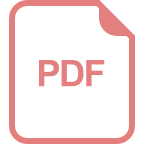
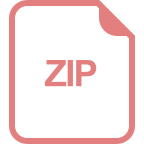
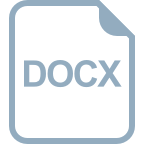
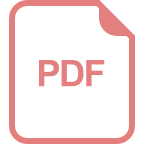
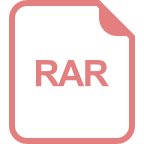