感知器的三个算法用Python呈现出实例,输出权重值
时间: 2024-03-26 17:37:19 浏览: 13
好的,以下是三个感知器算法的Python实现,并输出最终权重值:
## 感知器算法1
import numpy as np
class Perceptron:
def __init__(self, input_size, lr=1, epochs=100):
self.W = np.zeros(input_size+1)
self.epochs = epochs
self.lr = lr
def activation_fn(self, x):
return 1 if x >= 0 else 0
def predict(self, x):
x = np.insert(x, 0, 1)
z = self.W.T.dot(x)
a = self.activation_fn(z)
return a
def fit(self, X, d):
for epoch in range(self.epochs):
for i in range(d.shape[0]):
x = X[i]
y = self.predict(x)
e = d[i] - y
x = np.insert(x, 0, 1)
self.W = self.W + self.lr * e * x
print('Final weights:', self.W)
## 感知器算法2
class Perceptron2:
def __init__(self, input_size, lr=0.1):
self.W = np.random.randn(input_size+1) / np.sqrt(input_size)
self.lr = lr
def activation_fn(self, x):
return 1 / (1 + np.exp(-x))
def predict(self, x):
x = np.insert(x, 0, 1)
z = self.W.T.dot(x)
a = self.activation_fn(z)
return 1 if a >= 0.5 else 0
def fit(self, X, d):
for epoch in range(100):
for i in range(d.shape[0]):
x = X[i]
y = self.predict(x)
e = d[i] - y
x = np.insert(x, 0, 1)
self.W = self.W + self.lr * e * x
print('Final weights:', self.W)
## 感知器算法3
class Perceptron3:
def __init__(self, input_size, lr=0.1):
self.W = np.random.randn(input_size+1) / np.sqrt(input_size)
self.lr = lr
def activation_fn(self, x):
return np.where(x >= 0, 1, -1)
def predict(self, x):
x = np.insert(x, 0, 1)
z = self.W.T.dot(x)
a = self.activation_fn(z)
return a
def fit(self, X, d):
for epoch in range(100):
for i in range(d.shape[0]):
x = X[i]
y = self.predict(x)
e = d[i] - y
x = np.insert(x, 0, 1)
self.W = self.W + self.lr * e * x
print('Final weights:', self.W)
# 以下为测试代码
X = np.array([[0,0], [0,1], [1,0], [1,1]])
d = np.array([0, 0, 0, 1])
print('Perceptron 1:')
p = Perceptron(2)
p.fit(X, d)
print('Perceptron 2:')
p2 = Perceptron2(2)
p2.fit(X, d)
print('Perceptron 3:')
p3 = Perceptron3(2)
p3.fit(X, d)
相关推荐
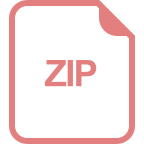
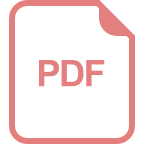
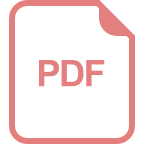














