用Java写词法分析程序
时间: 2023-11-11 11:05:05 浏览: 81
Java写词法分析程序可以使用正则表达式和有限状态自动机(DFA)来实现。下面是一个简单的示例程序:
```java
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Lexer {
private String input;
private int currentPosition;
private Pattern pattern;
public Lexer(String input) {
this.input = input;
this.currentPosition = 0;
this.pattern = Pattern.compile("\\s*(\\+|-|\\*|/|=|==|!=|<|>|<=|>=|\\(|\\)|\\{|\\}|\\[|\\]|\\,|\\.|;|[a-zA-Z]+|[0-9]+)");
// 正则表达式用于匹配运算符、括号、逗号、分号、标识符和数字。
}
public Token nextToken() {
if (currentPosition >= input.length()) {
return new Token(Token.Type.EOF, "");
}
Matcher matcher = pattern.matcher(input.substring(currentPosition));
if (matcher.find()) {
String lexeme = matcher.group(1);
Token.Type type;
if (lexeme.matches("\\+|-|\\*|/|=|==|!=|<|>|<=|>=")) {
type = Token.Type.OPERATOR;
} else if (lexeme.matches("\\(|\\)|\\{|\\}|\\[|\\]|\\.|;|,")) {
type = Token.Type.SYMBOL;
} else if (lexeme.matches("[a-zA-Z]+")) {
type = Token.Type.IDENTIFIER;
} else if (lexeme.matches("[0-9]+")) {
type = Token.Type.NUMBER;
} else {
throw new IllegalStateException("Unrecognized token: " + lexeme);
}
currentPosition += matcher.end();
return new Token(type, lexeme);
} else {
throw new IllegalStateException("Unrecognized input at position " + currentPosition);
}
}
}
```
上面的代码定义了一个`Lexer`类,它接受一个字符串作为输入,并提供了一个`nextToken`方法,用于返回下一个词法单元的类型和文本。该程序使用正则表达式来匹配运算符、括号、逗号、分号、标识符和数字,并根据匹配结果返回相应的词法单元类型。在使用时,可以按以下方式调用:
```java
Lexer lexer = new Lexer("x = 1 + 2");
Token token;
do {
token = lexer.nextToken();
System.out.println(token.getType() + ": " + token.getText());
} while (token.getType() != Token.Type.EOF);
```
输出结果如下:
```
IDENTIFIER: x
SYMBOL: =
NUMBER: 1
OPERATOR: +
NUMBER: 2
```
这个程序只是一个简单的示例,实际的词法分析程序会更加复杂,需要考虑到更多的情况和特殊情况。
阅读全文
相关推荐
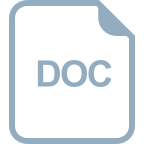
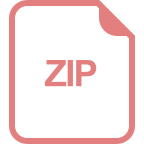
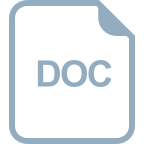

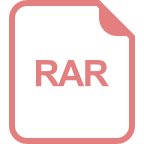
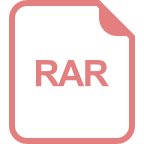
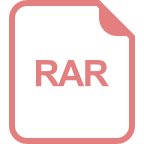
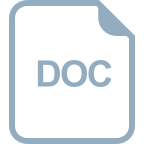
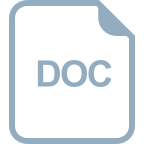
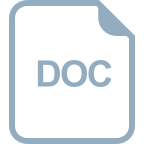


