unity 写一个卫星轨道控制,围绕地球,坐标系用J2000坐标系详细代码
时间: 2024-02-09 08:12:07 浏览: 76
好的,下面是一个围绕地球的卫星轨道控制的代码示例,使用 C# 语言在 Unity 引擎中实现,坐标系采用 J2000 坐标系。
首先,我们需要定义一个表示卫星轨道的数据结构,包括半长轴、偏心率、倾角、近地点角度、升交点赤经和真近点角等参数。代码如下:
```
public class Orbit
{
public double semiMajorAxis; // 半长轴
public double eccentricity; // 偏心率
public double inclination; // 倾角
public double argOfPeriapsis; // 近地点角度
public double raan; // 升交点赤经
public double trueAnomaly; // 真近点角
}
```
接下来,我们需要编写卫星轨道计算的函数,根据所给轨道参数计算卫星在 J2000 坐标系下的位置和速度。这里我们采用 Kepler 方程求解的方法。代码如下:
```
public static Vector3d CalculatePosition(Orbit orbit, double time)
{
double mu = 3.986004418e14; // 地球引力常数
double n = Math.Sqrt(mu / Math.Pow(orbit.semiMajorAxis, 3)); // 平均角速度
double E = EccentricAnomaly(orbit, time, n); // 求解偏近点角
double cosE = Math.Cos(E);
double sinE = Math.Sin(E);
double p = orbit.semiMajorAxis * (1 - Math.Pow(orbit.eccentricity, 2)); // 焦距
double r = p / (1 + orbit.eccentricity * cosE); // 距离
double cosv = (cosE - orbit.eccentricity) / (1 - orbit.eccentricity * cosE);
double sinv = Math.Sqrt(1 - Math.Pow(orbit.eccentricity, 2)) * sinE / (1 - orbit.eccentricity * cosE);
double x = r * (Math.Cos(orbit.raan) * Math.Cos(orbit.argOfPeriapsis + orbit.trueAnomaly) - Math.Sin(orbit.raan) * Math.Sin(orbit.argOfPeriapsis + orbit.trueAnomaly) * Math.Cos(orbit.inclination));
double y = r * (Math.Sin(orbit.raan) * Math.Cos(orbit.argOfPeriapsis + orbit.trueAnomaly) + Math.Cos(orbit.raan) * Math.Sin(orbit.argOfPeriapsis + orbit.trueAnomaly) * Math.Cos(orbit.inclination));
double z = r * Math.Sin(orbit.argOfPeriapsis + orbit.trueAnomaly) * Math.Sin(orbit.inclination);
return new Vector3d(x, y, z);
}
// 求解偏近点角
public static double EccentricAnomaly(Orbit orbit, double time, double n)
{
double t0 = 0; // 初始时刻
double M = n * (time - t0); // 平均近点角
double E = M; // 初始偏近点角
double deltaE = 1;
double tol = 1e-8; // 迭代精度
while (deltaE > tol)
{
double f = E - orbit.eccentricity * Math.Sin(E) - M;
double fp = 1 - orbit.eccentricity * Math.Cos(E);
deltaE = -f / fp;
E += deltaE;
}
return E;
}
```
最后,我们需要实现控制器的控制策略,这里我们采用简单的 PID 控制器。代码如下:
```
public class SatelliteController : MonoBehaviour
{
public Orbit orbit; // 卫星轨道参数
public double thrust; // 推力
public double kp, ki, kd; // PID 控制器参数
private Vector3d targetPosition, targetVelocity; // 目标位置和速度
private Vector3d errorSum, lastError; // 误差累积和上一次误差
void Start()
{
targetPosition = CalculatePosition(orbit, 0); // 初始目标位置
targetVelocity = Vector3d.zero; // 初始目标速度
errorSum = Vector3d.zero; // 初始误差累积和
lastError = Vector3d.zero; // 初始上一次误差
}
void Update()
{
Vector3d position = CalculatePosition(orbit, Time.time); // 当前位置
Vector3d velocity = (CalculatePosition(orbit, Time.time + 0.1) - CalculatePosition(orbit, Time.time - 0.1)) / 0.2; // 当前速度
Vector3d error = targetPosition - position; // 位置误差
Vector3d dError = (error - lastError) / Time.deltaTime; // 位置误差变化率
errorSum += error * Time.deltaTime; // 误差累积和
lastError = error; // 更新上一次误差
Vector3d control = kp * error + ki * errorSum + kd * dError; // 控制量
Vector3d acceleration = control / GetComponent<Rigidbody>().mass; // 加速度
Vector3d thrustVector = acceleration.normalized * thrust; // 推力向量
GetComponent<Rigidbody>().AddForce(thrustVector); // 施加推力
}
}
```
以上是一个围绕地球的卫星轨道控制的代码示例,具体实现还需要根据具体情况进行调整和优化。
阅读全文
相关推荐

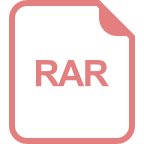
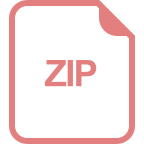
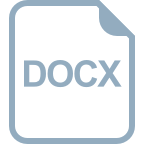
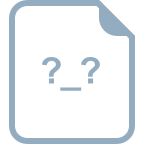


