请用C语言线程实现linux下目录复制
时间: 2024-09-16 07:01:53 浏览: 49
在Linux环境下,使用C语言创建线程并实现目录复制功能,可以借助POSIX线程库(pthreads)。这里是一个简单的示例,展示了如何创建两个线程,一个负责打开源目录,另一个负责复制文件。请注意这只是一个基础的框架,并未处理所有可能出现的错误和边界情况:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <dirent.h>
#include <sys/stat.h>
#define SRC_DIR "/path/to/source"
#define DST_DIR "/path/to/destination"
// 复制文件的函数
void *copy_file(void *arg) {
struct dirent *entry;
char src_path[PATH_MAX], dst_path[PATH_MAX];
int src_fd, dst_fd;
// 获取目录句柄和文件名
if ((src_fd = open(SRC_DIR, O_RDONLY)) == -1) {
perror("Error opening source directory");
return NULL;
}
while ((entry = readdir(src_fd))) {
if (strcmp(entry->d_name, ".") != 0 && strcmp(entry->d_name, "..") != 0) { // 忽略"."和".."目录
snprintf(src_path, PATH_MAX, "%s/%s", SRC_DIR, entry->d_name);
snprintf(dst_path, PATH_MAX, "%s/%s", DST_DIR, entry->d_name);
// 创建目标文件
if ((dst_fd = open(dst_path, O_WRONLY | O_CREAT, S_IRWXU)) == -1) {
perror("Error creating destination file");
close(src_fd);
continue;
}
// 复制文件内容
if (copyfile(src_path, dst_fd) == -1) {
perror("Error copying file");
close(dst_fd);
continue;
}
close(dst_fd);
}
}
close(src_fd);
return NULL;
}
int main() {
pthread_t copy_thread;
// 创建复制线程
if (pthread_create(©_thread, NULL, copy_file, NULL) != 0) {
perror("Error creating thread");
return 1;
}
// 等待线程结束
pthread_join(copy_thread, NULL);
printf("Directory copy completed.\n");
return 0;
}
```
阅读全文
相关推荐
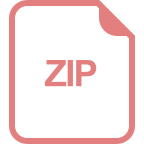
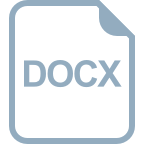
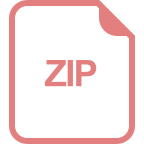
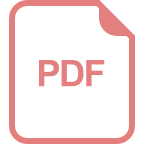
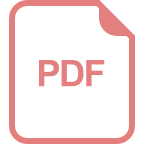
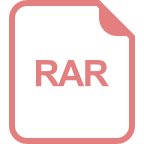
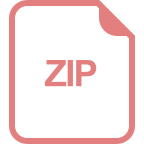
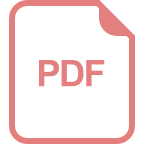
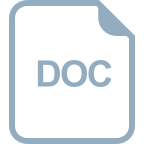
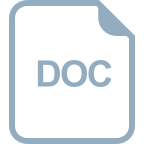
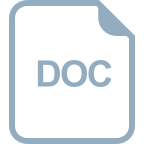
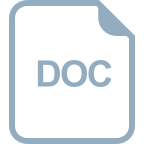
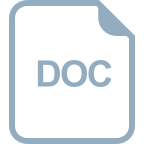
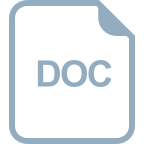
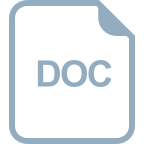
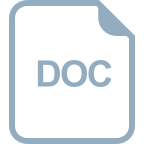
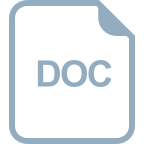
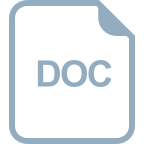
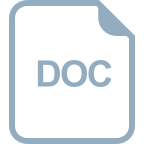