请使用redux编写一个demo
时间: 2024-03-27 07:36:47 浏览: 61
好的,以下是一个简单的 Redux Demo,它包括一个计数器组件和一个 Redux store:
1. 安装 Redux:在项目中使用 npm 或 yarn 安装 Redux。
`npm install redux`
2. 创建 store:使用 createStore() 方法创建一个 store,并将 reducer 作为参数传递给它。
```
import { createStore } from 'redux';
// 定义 reducer
const counterReducer = (state = 0, action) => {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
}
// 创建 store
const store = createStore(counterReducer);
```
3. 定义 reducer:reducer 是一个纯函数,接收当前状态和 action,返回新状态。它必须是纯函数,因为它不应该修改输入参数,也不应该有任何副作用。
4. 创建 action:action 是一个简单的对象,描述应用程序中发生的事件。它必须包含一个 type 属性,指定要执行的操作类型。
```
// 创建 action
const incrementAction = { type: 'INCREMENT' };
const decrementAction = { type: 'DECREMENT' };
```
5. 分发 action:使用 store.dispatch() 方法将 action 分发到 reducer 中。
```
// 分发 action
store.dispatch(incrementAction); // state = 1
store.dispatch(incrementAction); // state = 2
store.dispatch(decrementAction); // state = 1
```
6. 访问状态:使用 store.getState() 方法访问 store 中的当前状态。
```
// 访问状态
console.log(store.getState()); // 1
```
7. 监听变化:使用 store.subscribe() 方法监听 store 中状态的变化,并在状态发生变化时执行相应的操作。
```
// 监听变化
store.subscribe(() => console.log(store.getState()));
```
完整代码如下:
```
import { createStore } from 'redux';
// 定义 reducer
const counterReducer = (state = 0, action) => {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
}
// 创建 store
const store = createStore(counterReducer);
// 创建 action
const incrementAction = { type: 'INCREMENT' };
const decrementAction = { type: 'DECREMENT' };
// 分发 action
store.dispatch(incrementAction); // state = 1
store.dispatch(incrementAction); // state = 2
store.dispatch(decrementAction); // state = 1
// 访问状态
console.log(store.getState()); // 1
// 监听变化
store.subscribe(() => console.log(store.getState()));
```
阅读全文
相关推荐
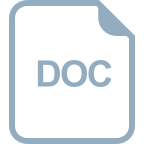
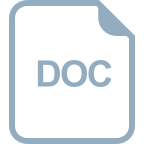
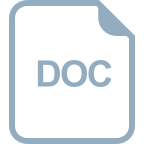
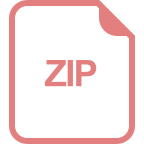
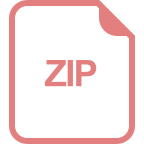
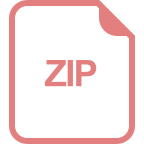
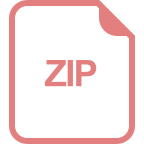
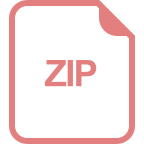
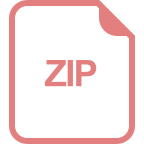
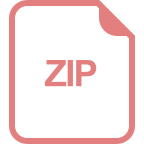
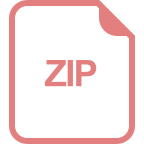
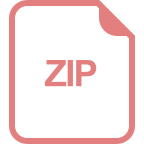
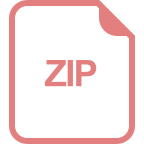
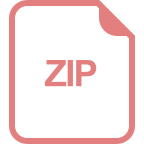
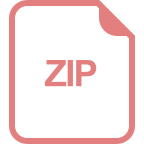
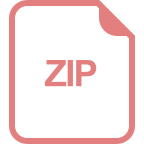
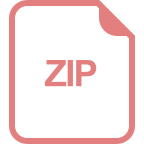

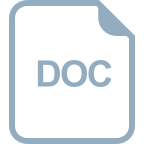