编程实现建立一个数据元素值是一个字符的单链表,并借助栈的入栈、出栈等操作判断此单链表中字符序列是否构成回文的操作。
时间: 2023-12-10 21:38:01 浏览: 34
这里给出C++实现的代码,具体思路是利用快慢指针找到单链表的中间节点,然后将后半段节点逆序并利用栈进行比较判断是否为回文。
```cpp
#include<iostream>
#include<stack>
using namespace std;
//定义单链表节点
struct Node{
char data;
Node *next;
Node(char c=0):data(c),next(NULL){}
};
//判断单链表是否为回文
bool isPalindrome(Node *head){
if(head == NULL) return false;
Node *slow = head, *fast = head; //快慢指针
stack<char> s; //定义栈
while(fast != NULL && fast->next != NULL){
s.push(slow->data);
slow = slow->next;
fast = fast->next->next;
}
//如果链表长度为奇数,跳过中间节点
if(fast != NULL) slow = slow->next;
while(slow != NULL){
if(s.top() != slow->data) return false;
s.pop();
slow = slow->next;
}
return true;
}
int main(){
//建立单链表
Node *head = new Node(), *p = head;
string str = "level";
for(char c : str){
Node *newNode = new Node(c);
p->next = newNode;
p = p->next;
}
//判断是否为回文
bool flag = isPalindrome(head->next);
if(flag) cout<<"是回文"<<endl;
else cout<<"不是回文"<<endl;
return 0;
}
```
相关推荐
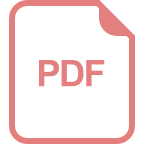
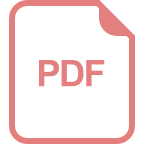
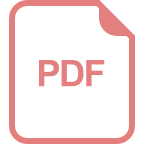














