utf8转cstring
时间: 2023-10-08 16:06:53 浏览: 77
UTF-8到C字符串的转换可以使用以下代码:
```cpp
#include <iostream>
#include <cstring>
int main()
{
std::string utf8Str = u8"Hello, 你好";
const char* cStr = utf8Str.c_str();
std::cout << "UTF-8字符串:" << utf8Str << std::endl;
std::cout << "转换后的C字符串:" << cStr << std::endl;
return 0;
}
```
这里使用了`std::string::c_str()`函数来得到C字符串,在使用之前可以将UTF-8字符串存储到std::string中。
相关问题
cstring 转utf8
将CString转换为UTF-8编码的字符串可以通过以下步骤完成:
1. 首先,确定CString的长度,可以通过调用CString的GetLength()函数获得。
2. 创建一个相应大小的数组来存储UTF-8编码的字符串。
3. 使用WideCharToMultiByte函数将CString转换为多字节字符串。该函数提供了将宽字符转换为多字节字符的功能,并且可以指定编码类型。
以下是一个示例代码:
```cpp
#include <atlstr.h>
#include <Windows.h>
CString CStringToUTF8(const CString& cstr)
{
int utf8Length = WideCharToMultiByte(CP_UTF8, 0, cstr, cstr.GetLength(), NULL, 0, NULL, NULL);
char* utf8Buffer = new char[utf8Length + 1];
WideCharToMultiByte(CP_UTF8, 0, cstr, cstr.GetLength(), utf8Buffer, utf8Length, NULL, NULL);
utf8Buffer[utf8Length] = '\0';
CString utf8Str(utf8Buffer);
delete[] utf8Buffer;
return utf8Str;
}
```
使用示例:
```cpp
CString cstr(_T("你好,世界!")); // 需要转换的CString
CString utf8Str = CStringToUTF8(cstr); // 转换为UTF-8编码的CString
AfxMessageBox(utf8Str); // 弹出消息框显示UTF-8编码的字符串
```
以上代码将CString对象cstr转换为UTF-8编码的字符串utf8Str,并通过消息框显示出来。
Cstring 转 utf-8
`CString` 是 MFC(Microsoft Foundation Classes)库中的一种字符串类型,在 Windows 应用程序开发中常用于处理文本数据。如果你想要将 `CString` 对象转换为 UTF-8 格式,首先需要确保 `CString` 中存储的是可以编码成 UTF-8 的字符。
下面是一个简单的步骤概述:
1. 确保 `CString` 内部的数据是宽字符(如 `wchar_t*` 或 `TCHAR*`),因为 `MFC` 中的默认字符集通常是多字节字符系统(MBCS),它并不直接支持 UTF-8。
2. 如果你需要把宽字符转换为 `char*` 或 `std::string`(UTF-8),你可以使用 `AtoWideChar` 和 `WideCharToMultiByte` 函数来进行转换。但是请注意,Windows API 提供的这些函数通常用于从 ANSI 到 Unicode,或从 Unicode 到 ANSI 的转换,并不是针对 UTF-8 的直接转换。
3. 如果 `CString` 实际上已经是 UTF-8 编码的,你可以直接将其转换为 `std::string` 或者复制到一个 `char*` 后,声明为 UTF-8 字符串。
```cpp
// 示例
CString utf8Str;
// 假设已经填充了UTF-8字符串
// 将CString转换为std::string,假设内部是utf-8编码
std::string utf8String = utf8Str.GetString();
// 或者复制到一个新的char*变量
char* utf8Chars = new char[utf8Str.GetLength()];
memcpy(utf8Chars, utf8Str, utf8Str.GetLength());
```
阅读全文
相关推荐
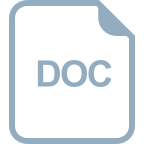
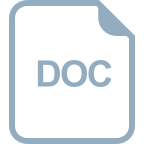
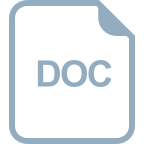
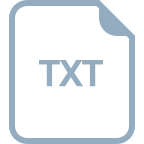
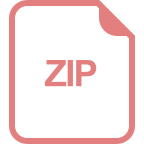


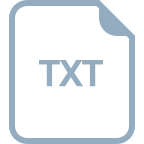








