c语言列举所有输出铃声代码举例?
时间: 2023-12-14 17:03:44 浏览: 32
以下是一个简单的 C 语言程序,用于输出铃声:
```
#include <stdio.h>
#include <windows.h>
int main()
{
printf("\a");
Sleep(1000); // 延迟一秒钟
printf("\a");
return 0;
}
```
其中 `\a` 是输出铃声的转义字符。运行这个程序后会听到两个铃声。
相关问题
c语言输出所有铃声代码举例?
可以使用以下代码来输出所有铃声代码:
#include <stdio.h>
int main() {
for(int i=0; i<256; i++) {
printf("code %d: \a\n", i);
}
return 0;
}
这段代码会输出从0到255的所有铃声代码。
c语言播放系统默认铃声的代码举例?
以下是C语言播放系统默认铃声的代码举例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <Windows.h>
int main()
{
printf("Playing default system beep...\n");
Beep(750, 300);
return 0;
}
```
这段代码使用了Windows API中的Beep函数,播放系统默认的铃声。在Beep函数中,第一个参数是频率,第二个参数是时长,可根据需要自行修改。
相关推荐
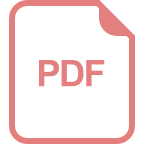
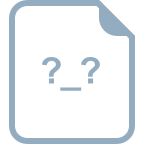












