七牛云图片上传html
时间: 2024-02-20 17:50:01 浏览: 147
以下是基于七牛云的图片上传的HTML代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>图片上传</title>
<script src="https://cdn.staticfile.org/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.staticfile.org/plupload/2.1.9/plupload.full.min.js"></script>
<script src="https://cdn.staticfile.org/qiniu-js/1.0.27/qiniu.min.js"></script>
</head>
<body>
<div id="container">
<a href="javascript:;" class="btn btn-primary" id="pickfiles">选择文件</a>
<a href="javascript:;" class="btn btn-primary" id="uploadfiles">上传文件</a>
</div>
<table class="table table-striped table-hover">
<thead>
<tr>
<th>文件名</th>
<th>大小</th>
<th>状态</th>
<th>链接</th>
</tr>
</thead>
<tbody id="fsUploadProgress">
</tbody>
</table>
<script type="text/javascript">
var uploader = Qiniu.uploader({
runtimes: 'html5,flash,html4',
browse_button: 'pickfiles',
container: 'container',
drop_element: 'container',
flash_swf_url: 'https://cdn.staticfile.org/plupload/2.1.9/Moxie.swf',
dragdrop: true,
chunk_size: '4mb',
multi_selection: !(moxie.core.utils.Env.OS.toLowerCase() === "ios"),
uptoken_url: '/uptoken', // ajax请求uptoken的Url,强烈建议设置(服务端提供)
domain: '你的七牛空间域名',
get_new_uptoken: false,
auto_start: true,
log_level: 5,
init: {
'FilesAdded': function(up, files) {
plupload.each(files, function(file) {
var progress = new FileProgress(file, 'fsUploadProgress');
progress.setStatus("等待...");
progress.bindUploadCancel(up);
});
},
'BeforeUpload': function(up, file) {
var progress = new FileProgress(file, 'fsUploadProgress');
var chunk_size = plupload.parseSize(this.getOption('chunk_size'));
if (up.runtime === 'html5' && chunk_size) {
progress.setChunkProgess(chunk_size);
}
},
'UploadProgress': function(up, file) {
var progress = new FileProgress(file, 'fsUploadProgress');
var chunk_size = plupload.parseSize(this.getOption('chunk_size'));
progress.setProgress(file.percent + "%", file.speed, chunk_size);
},
'UploadComplete': function() {
$('#success').show();
},
'FileUploaded': function(up, file, info) {
var progress = new FileProgress(file, 'fsUploadProgress');
progress.setComplete(up, info);
},
'Error': function(up, err, errTip) {
var progress = new FileProgress(err.file, 'fsUploadProgress');
progress.setError();
progress.setStatus(errTip);
}
}
});
function FileProgress(file, targetID) {
this.fileProgressID = file.id;
this.opacity = 100;
this.height = 0;
this.fileSize = plupload.formatSize(file.size);
this.fileUploaded = 0;
this.speed = "";
this.timestamp = new Date().getTime();
this.targetID = targetID;
this.fileProgressWrapper = $('#' + this.fileProgressID);
if (!this.fileProgressWrapper.length) {
var html = '<tr id="' + this.fileProgressID + '">';
html += '<td>' + file.name + '</td><td>' + this.fileSize + '</td><td class="status">等待...</td><td class="url"></td>';
html += '</tr>';
this.fileProgressWrapper = $(html);
$('#' + this.targetID).append(this.fileProgressWrapper);
}
}
FileProgress.prototype.setProgress = function(progress, speed, chunk_size) {
var fileProgress = $('#' + this.fileProgressID);
this.fileUploaded = progress;
this.speed = speed;
var now = new Date().getTime();
var diff = now - this.timestamp;
if (diff < 1000) {
diff = 1000;
}
var uploaded = this.fileUploaded;
if (uploaded && uploaded > 0) {
var uploadSpeed = uploaded / diff * 1000;
var timeLeft = Math.ceil((100 - uploaded) / uploaded * diff / 1000);
var chunksInfo = '';
if (chunk_size) {
var totalChunks = Math.ceil(file.size / chunk_size);
var chunkIndex = Math.ceil(uploaded / chunk_size);
chunksInfo = ' - ' + chunkIndex + '/' + totalChunks;
}
fileProgress.find('.status').html('上传中' + chunksInfo);
fileProgress.find('.url').html('');
fileProgress.find('.progress').show().find('.progress-bar').css('width', uploaded + '%');
fileProgress.find('.info').html(plupload.formatSize(uploadSpeed) + '/s | 预计剩余时间:' + timeLeft + 's');
}
};
FileProgress.prototype.setComplete = function(up, info) {
var fileProgress = $('#' + this.fileProgressID);
fileProgress.find('.status').html("上传成功");
var domain = up.getOption('domain');
var res = $.parseJSON(info);
var sourceLink = 'http://' + domain + '/' + res.key;
fileProgress.find('.url').html('<a href="' + sourceLink + '" target="_blank">' + sourceLink + '</a>');
};
FileProgress.prototype.setError = function() {
var fileProgress = $('#' + this.fileProgressID);
fileProgress.find('.status').html("上传失败");
fileProgress.find('.url').html('');
};
FileProgress.prototype.setStatus = function(status) {
var fileProgress = $('#' + this.fileProgressID);
fileProgress.find('.status').html(status);
fileProgress.find('.url').html('');
};
FileProgress.prototype.setChunkProgess = function(chunk_size) {
var fileProgress = $('#' + this.fileProgressID);
var totalChunks = Math.ceil(fileProgress.find('.progress').width() / chunk_size);
for (var i = 0; i < totalChunks; i++) {
fileProgress.find('.progress').append('<div class="progress-chunk"></div>');
}
};
FileProgress.prototype.bindUploadCancel = function(up) {
var self = this;
$('#' + this.fileProgressID + ' .uploadCancel').click(function() {
up.removeFile(self.file);
$('#' + self.fileProgressID).remove();
});
};
</script>
</body>
</html>
```
需要注意以下几点:
1. 需要引入jQuery、plupload和qiniu-js的相关JS文件。
2. 在`Qiniu.uploader()`的参数中,需要根据实际情况设置`uptoken_url`和`domain`,其中`uptoken_url`是用于获取上传凭证的地址,`domain`是你的七牛空间域名。
3. 上传的进度和状态会在页面上实时显示,需要在`init`参数中对上传过程的回调进行处理。
阅读全文
相关推荐
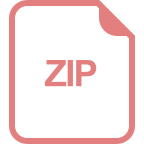
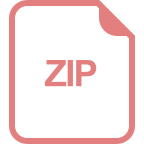
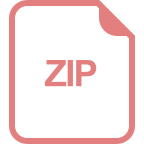
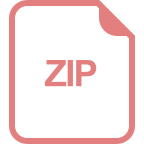
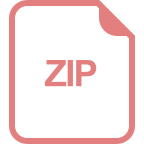
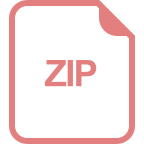
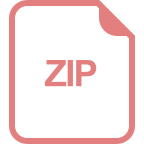
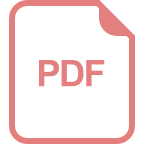
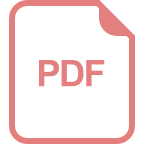
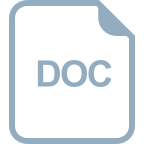
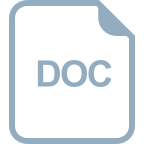
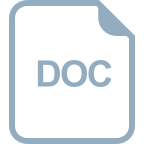
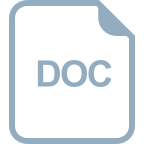
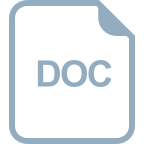

