java框架 卡斯卡_zookeeper sasl身份验证问题
时间: 2023-10-26 12:27:03 浏览: 65
在使用Java框架中的ZooKeeper时,如果需要进行SASL身份验证,可以按照以下步骤进行操作:
1.在ZooKeeper服务器端配置JVM参数,启用SASL身份验证,例如:
```
-Dzookeeper.serverCnxnFactory=org.apache.zookeeper.server.NettyServerCnxnFactory
-Djava.security.auth.login.config=/path/to/zookeeper_server_jaas.conf
```
2.在ZooKeeper客户端端配置JVM参数,启用SASL身份验证,例如:
```
-Djava.security.auth.login.config=/path/to/zookeeper_client_jaas.conf
```
3.在ZooKeeper服务器端和客户端端分别编写对应的JAAS配置文件,例如:
服务器端配置文件zookeeper_server_jaas.conf:
```
Server {
org.apache.zookeeper.server.auth.DigestLoginModule required
user_admin="password";
};
```
客户端配置文件zookeeper_client_jaas.conf:
```
Client {
org.apache.zookeeper.server.auth.DigestLoginModule required
username="admin"
password="password";
};
```
其中,user_admin和password是服务器端的用户名和密码,username和password是客户端的用户名和密码。
4.在ZooKeeper客户端端编写对应的代码,例如:
```
import org.apache.zookeeper.*;
import org.apache.zookeeper.data.Stat;
import java.io.IOException;
import java.util.concurrent.CountDownLatch;
public class ZooKeeperClient {
private static final String CONNECT_STRING = "localhost:2181";
private static final int SESSION_TIMEOUT = 5000;
private static final String ZOOKEEPER_PATH = "/test";
private static CountDownLatch countDownLatch = new CountDownLatch(1);
public static void main(String[] args) throws IOException, InterruptedException, KeeperException {
ZooKeeper zooKeeper = new ZooKeeper(CONNECT_STRING, SESSION_TIMEOUT, new Watcher() {
@Override
public void process(WatchedEvent event) {
if (event.getState() == Event.KeeperState.SyncConnected) {
countDownLatch.countDown();
}
}
});
countDownLatch.await();
String result = new String(zooKeeper.getData(ZOOKEEPER_PATH, false, new Stat()));
System.out.println(result);
zooKeeper.close();
}
}
```
以上代码中,我们使用了CountDownLatch来等待ZooKeeper客户端连接上ZooKeeper服务器。在连接成功之后,我们调用了zooKeeper.getData()方法来获取指定路径节点的数据。
以上就是在Java框架中使用ZooKeeper进行SASL身份验证的方法。
相关推荐
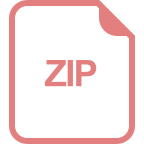

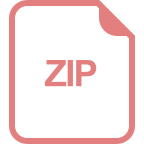
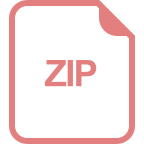
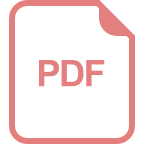
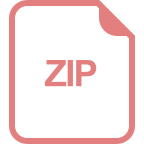
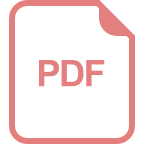
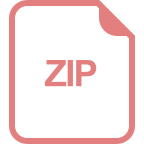
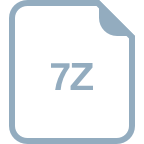
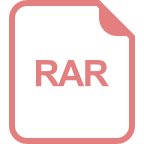
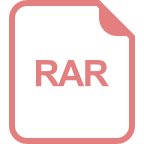
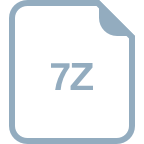
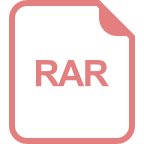
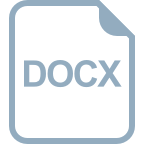
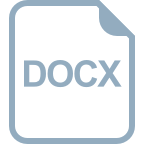