plot->graph()->setName(expression);这个代码有什么用
时间: 2024-01-24 12:17:59 浏览: 167
这段代码是用于设置图表中曲线的名称。具体来说,它的作用是:
1. `plot`是一个QCustomPlot对象,表示图表中的绘图区域;
2. `graph()`是QCustomPlot对象的函数,返回当前绘图区域中最后一个添加的曲线对象;
3. `setName(expression)`是QCPGraph对象的函数,用于设置曲线的名称,其中`expression`表示曲线的名称字符串。
综上所述,这段代码的作用是为当前绘图区域中最后一个添加的曲线对象设置名称为`expression`。这样,在图表中就可以方便地识别出每条曲线所代表的数据。
相关问题
QString expression = ui->expressionEdit->text(); if (expression.isEmpty()) return; QCustomPlot *plot = ui->plotWidget; plot->addGraph(); plot->graph()->setPen(QPen(Qt::blue)); plot->graph()->setName(expression); double xMin = -10, xMax = 10, yMin = -10, yMax = 10; plot->xAxis->setRange(xMin, xMax); plot->yAxis->setRange(yMin, yMax); QVector<double> xValues, yValues;解释这段代码的逻辑
这段代码的逻辑是在一个Qt界面应用程序中,当用户在expressionEdit文本框中输入了一个数学表达式后,将该表达式的图像绘制到名为plotWidget的QCustomPlot对象上。
首先,程序检查expressionEdit文本框中是否有内容。如果没有内容,则直接返回。
接下来,程序创建一个新的曲线图,并将其添加到plotWidget对象中。该曲线图的线条颜色被设置为蓝色。
接着,程序为该曲线图设置一个名称,该名称为用户在expressionEdit文本框中输入的表达式。
然后,程序设置了x轴和y轴的范围为-10到10。
最后,程序计算了x和y值的向量,并将它们存储在xValues和yValues中,以便绘制该曲线图的图像。
详细地教我用Qt制作一个函数图像绘制器,要求能够输入任意函数,点击设置好的ok键后,把输入的函数转化成函数图像并在坐标轴中呈现出来,,生成的函数图像可以叠加,有一个清除键可以清楚之前生成的所有函数图像,有一个保存键可以保存当前的函数图像并且可以选择保存地址,转化为jpg图片文件
好的,下面是一个简单的Qt程序,可以实现你的需求。在这个程序中,我们使用了QCustomPlot库来实现函数图像的绘制。首先,需要在.pro文件中添加QCustomPlot库的路径:
```
INCLUDEPATH += /path/to/qcustomplot
```
然后,在主窗口的.ui文件中添加一个QCustomPlot控件,并添加两个按钮,一个用于添加函数图像,一个用于清除所有图像。代码如下:
mainwindow.ui:
```
<?xml version="1.0" encoding="UTF-8"?>
<ui version="4.0">
<class>MainWindow</class>
<widget class="QMainWindow" name="MainWindow">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>640</width>
<height>480</height>
</rect>
</property>
<property name="windowTitle">
<string>Function Plotter</string>
</property>
<widget class="QWidget" name="centralwidget">
<widget class="QCustomPlot" name="plot">
<geometry>
<rect>
<x>10</x>
<y>10</y>
<<width>620</width>
<height>400</height>
</rect>
</geometry>
</widget>
<widget class="QPushButton" name="addButton">
<property name="text">
<string>Add Function</string>
</property>
<geometry>
<rect>
<x>10</x>
<y>420</y>
<width>120</width>
<height>30</height>
</rect>
</geometry>
</widget>
<widget class="QPushButton" name="clearButton">
<property name="text">
<string>Clear All</string>
</property>
<geometry>
<rect>
<x>140</x>
<y>420</y>
<width>120</width>
<height>30</height>
</rect>
</geometry>
</widget>
</widget>
<widget class="QMenuBar" name="menubar">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>640</width>
<height>21</height>
</rect>
</property>
</widget>
<widget class="QStatusBar" name="statusbar"/>
</widget>
<resources/>
<connections/>
</ui>
```
接下来,在mainwindow.cpp中实现添加和清除图像的功能。首先,需要在头文件中包含QCustomPlot库的头文件和一些Qt头文件:
mainwindown.h:
```
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include "qcustomplot.h"
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void on_addButton_clicked();
void on_clearButton_clicked();
private:
Ui::MainWindow *ui;
QVector<double> x, y;
QCPGraph *graph;
};
#endif // MAINWINDOW_H
```
然后,在cpp文件中实现槽函数:
mainwindow.cpp:
```
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 设置坐标轴范围
ui->plot->xAxis->setRange(-10, 10);
ui->plot->yAxis->setRange(-10, 10);
// 添加坐标轴标签
ui->plot->xAxis->setLabel("x");
ui->plot->yAxis->setLabel("y");
// 添加网格线
ui->plot->setInteractions(QCP::iRangeDrag | QCP::iRangeZoom | QCP::iSelectPlottables);
ui->plot->xAxis->grid()->setSubGridVisible(true);
ui->plot->yAxis->grid()->setSubGridVisible(true);
ui->plot->xAxis->grid()->setZeroLinePen(Qt::NoPen);
ui->plot->yAxis->grid()->setZeroLinePen(Qt::NoPen);
ui->plot->xAxis->grid()->setPen(QPen(QColor(130, 130, 130), 0, Qt::DotLine));
ui->plot->yAxis->grid()->setPen(QPen(QColor(130, 130, 130), 0, Qt::DotLine));
ui->plot->xAxis->grid()->setSubGridPen(QPen(QColor(200, 200, 200), 0, Qt::DotLine));
ui->plot->yAxis->grid()->setSubGridPen(QPen(QColor(200, 200, 200), 0, Qt::DotLine));
// 初始化变量
graph = nullptr;
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::on_addButton_clicked()
{
// 获取函数表达式
QString expression = QInputDialog::getText(this, "Input Function", "Enter a function:");
// 解析函数表达式
x.clear();
y.clear();
for (double i=-10; i<=10; i+=0.1) {
QString str = expression;
str.replace("x", QString::number(i));
QScriptEngine engine;
QScriptValue result = engine.evaluate(str);
y.append(result.toNumber());
x.append(i);
}
// 绘制函数图像
if (graph == nullptr) {
graph = ui->plot->addGraph();
graph->setPen(QPen(QColor(255, 0, 0), 2));
}
graph->setData(x, y);
ui->plot->replot();
}
void MainWindow::on_clearButton_clicked()
{
// 清除所有图像
ui->plot->clearGraphs();
ui->plot->replot();
graph = nullptr;
}
```
最后,实现保存图像的功能。在mainwindow.cpp中添加一个槽函数,用于将绘制的函数图像保存为jpg文件:
```
void MainWindow::on_saveButton_clicked()
{
// 获取保存路径
QString filename = QFileDialog::getSaveFileName(this, tr("Save File"),
"",
tr("JPEG (*.jpg)"));
// 保存图像
if (!filename.isEmpty()) {
ui->plot->saveJpg(filename, 0, 0, 1.0, -1, 100);
}
}
```
在主窗口中添加一个保存按钮,并连接到这个槽函数:
mainwindow.ui:
```
<widget class="QPushButton" name="saveButton">
<property name="text">
<string>Save Image</string>
</property>
<geometry>
<rect>
<x>270</x>
<y>420</y>
<width>120</width>
<height>30</height>
</rect>
</geometry>
</widget>
```
mainwindow.cpp:
```
connect(ui->saveButton, &QPushButton::clicked, this, &MainWindow::on_saveButton_clicked);
```
完整的代码如下:
阅读全文
相关推荐
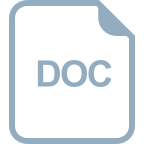
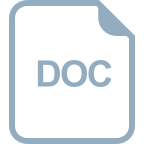
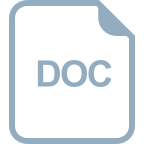
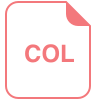
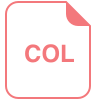
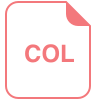
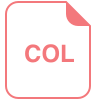
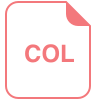
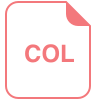
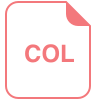
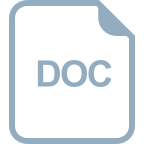
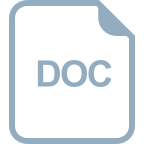
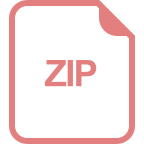
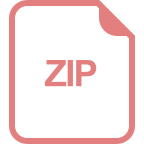
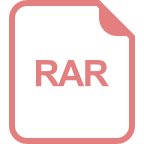
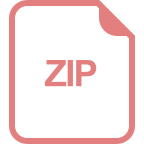