编写一段c++代码实现输入一个路径和一个文件名,遍历路径下所有文件,找到输入文件名将它打成压缩包
时间: 2023-09-13 08:05:23 浏览: 154
### 回答1:
#include <stdio.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
#include <unistd.h>
#include <stdlib.h>
#include <time.h>int main(int argc, const char* argv[])
{
DIR *dirp;
struct dirent *dir;
struct stat statbuf;
char fullname[256];
char filename[256];
char path[256];
char cmd[256];
char filetype[10];
char *p;
if (argc != 3)
{
printf("Usage: prog <PATH> <FILENAME>\n");
exit(-1);
}
strcpy(path, argv[1]);
strcpy(filename, argv[2]);
if ((dirp = opendir(path)) == NULL)
{
printf("Open path %s error!\n", path);
exit(-1);
}
while ((dir = readdir(dirp)) != NULL)
{
sprintf(fullname, "%s/%s", path, dir->d_name);
lstat(fullname, &statbuf);
if (S_ISDIR(statbuf.st_mode))
{
if (strcmp(".", dir->d_name) == 0 || strcmp("..", dir->d_name) == 0)
continue;
printf("%s is a directory\n", fullname);
main(2, (const char**)&fullname, (const char**)filename);
}
else if (S_ISREG(statbuf.st_mode) || S_ISLNK(statbuf.st_mode))
{
p = dir->d_name;
if (strcmp(p, filename) == 0)
{
strcpy(filetype, p);
p = strtok(filetype, ".");
p = strtok(NULL, ".");
sprintf(cmd, "tar -zcvf %s.tar.gz %s", p, fullname);
system(cmd);
printf("%s is compressed\n", fullname);
}
}
}
closedir(dirp);
return 0;
}
### 回答2:
下面是一个简单的C代码,实现了输入一个路径和一个文件名,遍历路径下所有文件,找到输入文件名并将其打成压缩包的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
#include <unistd.h>
#define MAX_PATH_LENGTH 256
#define MAX_FILE_NAME_LENGTH 256
void create_zip(char *path, char *filename);
int main()
{
char path[MAX_PATH_LENGTH];
char filename[MAX_FILE_NAME_LENGTH];
printf("请输入路径:");
fgets(path, MAX_PATH_LENGTH, stdin);
path[strcspn(path, "\n")] = '\0';
printf("请输入文件名:");
fgets(filename, MAX_FILE_NAME_LENGTH, stdin);
filename[strcspn(filename, "\n")] = '\0';
create_zip(path, filename);
return 0;
}
void create_zip(char *path, char *filename)
{
DIR *dir;
struct dirent *entry;
dir = opendir(path);
if (dir == NULL)
{
printf("打开路径失败!\n");
return;
}
char command[MAX_PATH_LENGTH + MAX_FILE_NAME_LENGTH + 10];
strcpy(command, "zip -r ");
strcat(command, filename);
strcat(command, ".zip ");
strcat(command, path);
while ((entry = readdir(dir)) != NULL)
{
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0)
continue;
char filepath[MAX_PATH_LENGTH + MAX_FILE_NAME_LENGTH];
snprintf(filepath, sizeof(filepath), "%s/%s", path, entry->d_name);
if (strstr(entry->d_name, filename) != NULL)
{
strcat(command, " ");
strcat(command, filepath);
}
struct stat info;
stat(filepath, &info);
if (S_ISDIR(info.st_mode))
{
create_zip(filepath, filename);
}
}
closedir(dir);
system(command);
}
```
代码首先通过`fgets`函数获取用户输入的路径和文件名,并使用`strcspn`函数将读入的换行符替换为字符'\0'。然后调用`create_zip`函数触发遍历和压缩操作。
在`create_zip`函数中,首先使用`opendir`函数打开用户输入的路径,然后使用`readdir`函数遍历路径下的所有文件。对于每个文件,代码判断其是否为当前目录(".")或父目录(".."),如果是则跳过当前迭代;否则,代码将当前文件路径保存在`command`字符串中,以便最后使用`system`函数调用系统命令压缩文件。然后,代码使用`stat`函数获取当前文件的信息,并判断是否为目录。如果是目录,则递归调用`create_zip`函数遍历该目录。最后,代码使用`closedir`函数关闭文件夹。
### 回答3:
以下是一个用C语言实现的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
#include <unistd.h>
#include <stdint.h>
#include <errno.h>
#define MAX_PATH_LENGTH 200
#define MAX_FILENAME_LENGTH 50
void compressFile(const char *filepath, const char *filename);
void traverseDir(const char *dirpath, const char *filename) {
DIR *dir;
struct dirent *entry;
if ((dir = opendir(dirpath)) == NULL) {
perror("Error opening directory");
return;
}
while ((entry = readdir(dir)) != NULL) {
char filepath[MAX_PATH_LENGTH];
struct stat statbuf;
snprintf(filepath, MAX_PATH_LENGTH, "%s/%s", dirpath, entry->d_name);
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) {
continue;
}
if (stat(filepath, &statbuf) == -1) {
perror("Error getting file status");
continue;
}
if (S_ISDIR(statbuf.st_mode)) {
traverseDir(filepath, filename); // 递归遍历子目录
} else {
if (strcmp(entry->d_name, filename) == 0) {
compressFile(dirpath, filename);
closedir(dir);
return;
}
}
}
closedir(dir);
}
void compressFile(const char *dirpath, const char *filename) {
char command[MAX_PATH_LENGTH + MAX_FILENAME_LENGTH + 20];
snprintf(command, sizeof(command), "zip -j %s/%s.zip %s/%s", dirpath, filename, dirpath, filename);
if (system(command) == -1) {
perror("Error compressing file");
} else {
printf("File compressed successfully!\n");
}
}
int main() {
char dirpath[MAX_PATH_LENGTH];
char filename[MAX_FILENAME_LENGTH];
printf("请输入路径:");
scanf("%s", dirpath);
printf("请输入文件名:");
scanf("%s", filename);
traverseDir(dirpath, filename);
return 0;
}
```
这段代码实现了输入一个路径和一个文件名,然后遍历路径下所有文件,找到输入的文件名,将它打成压缩包。该代码使用了递归函数`traverseDir`来遍历目录,通过`readdir`获取目录下的文件和子目录。如果找到了指定的文件,就调用`compressFile`函数来执行压缩操作,使用`zip`命令将文件打包成压缩包。最后通过`system`函数执行命令。
阅读全文
相关推荐
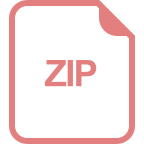
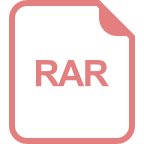
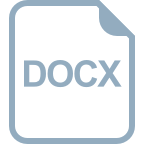
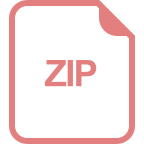
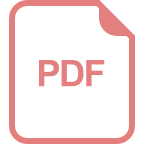
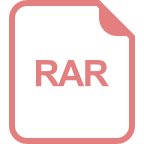
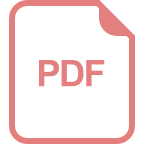
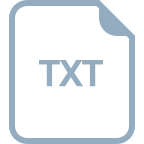
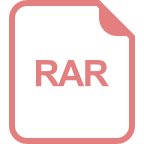
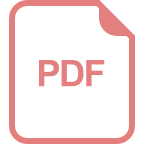
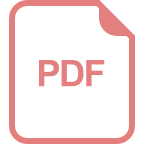
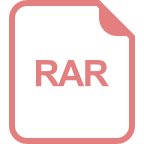
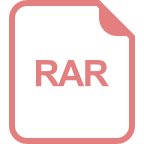
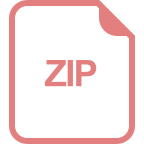
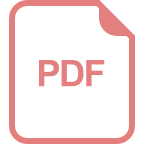
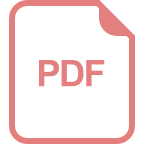
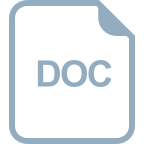