类图与Java代码转换举例
时间: 2024-09-28 14:04:04 浏览: 37
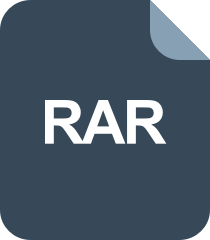
疯狂JAVA讲义

类图是一种可视化工具,它帮助软件开发者理解系统的架构和组件之间的关系。当设计类图时,通常会涉及类、接口、继承、封装和聚合等概念。以下是将类图转换成简单的Java代码的一个例子:
**类图示例:**
- 客户端(Client)
- 服务器(Server)
- 消息(Message)
- 通信协议(CommunicationProtocol)
对应的Java代码可能会如下所示:
```java
// 客户端类
class Client {
private String clientId;
private CommunicationProtocol protocol;
public Client(String clientId, CommunicationProtocol protocol) {
this.clientId = clientId;
this.protocol = protocol;
}
// 发送消息
public void sendMessage(Message message) {
protocol.sendMessage(message, this);
}
}
// 服务器类
class Server {
private String serverId;
private List<Client> clients;
public Server(String serverId) {
this.serverId = serverId;
clients = new ArrayList<>();
}
// 接收并处理消息
public void handleReceivedMessage(Message message) {
// 处理逻辑...
}
// 添加客户端
public void addClient(Client client) {
clients.add(client);
}
}
// 消息类
abstract class Message {
protected String content;
// 构造函数、getter和setter省略
abstract void sendMessage(Server sender);
}
// 通信协议接口
interface CommunicationProtocol {
void sendMessage(Message message, Object sender);
}
```
这只是一个基础的示例,实际项目中可能还需要考虑更多细节,比如异常处理、线程安全等问题。类图可以作为设计阶段的蓝图,而Java代码则是实现该蓝图的具体实现。
阅读全文
相关推荐
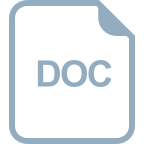
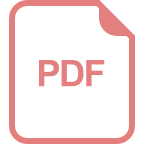

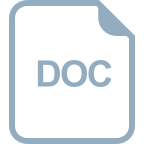
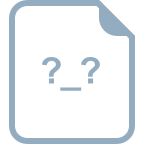
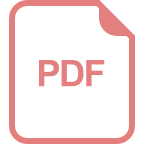
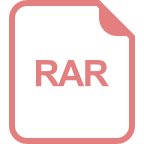
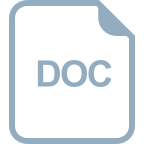
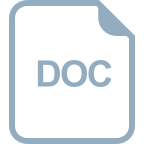
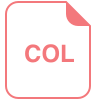
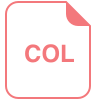
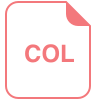
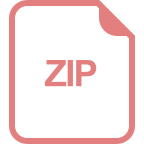
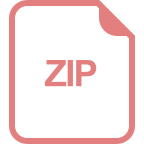
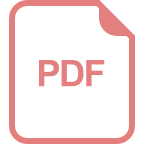
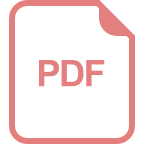