数据加密标准des实现c语言
时间: 2024-09-13 21:06:48 浏览: 49
DES (Data Encryption Standard) 是一种对称密钥加密算法,用于保护数据隐私。在C语言中实现DES通常会涉及以下几个步骤:
1. **包括头文件**:首先需要包含`openssl/des.h`头文件,它包含了DES函数库。
```c
#include <openssl/des.h>
```
2. **初始化 DES 结构体**:创建一个`DES_CTX`结构体,并使用`DES_set_key()`设置秘钥。
```c
DES_CTX *ctx;
DES_key_schedule key;
unsigned char key_bytes[8]; // 64位密钥
... // 设置key_bytes为秘钥
ctx = DES_new();
DES_set_key(ctx, key_bytes);
```
3. **加密/解密函数**:使用`DES_encrypt()`或`DES_decrypt()`函数进行操作。例如加密:
```c
unsigned char input[8], output[8];
... // 输入数据
DES_crypt(input, output, ctx); // 输出加密后的数据
```
4. **清理**:最后别忘了释放资源。
```c
DES_free_key(&key);
DES_free(ctx);
```
相关问题
DES加密代码实现C语言
以下是简单的 DES 加密和解密 C 代码实现:
```
#include <stdio.h>
#include <string.h>
#include <openssl/des.h>
#define BLOCK_SIZE 8
void pad(char *text) {
// 用空格填充文本,使其长度为 8 的倍数
int len = strlen(text);
while (len % BLOCK_SIZE != 0) {
text[len] = ' ';
len++;
}
}
void encrypt(char *key, char *plaintext, char *encrypted_text) {
// DES 加密
DES_key_schedule key_schedule;
DES_cblock key_des;
pad(plaintext);
DES_string_to_key(key, &key_des);
DES_set_key_checked(&key_des, &key_schedule);
for (int i = 0; i < strlen(plaintext); i += BLOCK_SIZE) {
DES_cblock plaintext_block, ciphertext_block;
memcpy(plaintext_block, plaintext + i, BLOCK_SIZE);
DES_ecb_encrypt(&plaintext_block, &ciphertext_block, &key_schedule, DES_ENCRYPT);
memcpy(encrypted_text + i, ciphertext_block, BLOCK_SIZE);
}
}
void decrypt(char *key, char *ciphertext, char *decrypted_text) {
// DES 解密
DES_key_schedule key_schedule;
DES_cblock key_des;
DES_string_to_key(key, &key_des);
DES_set_key_checked(&key_des, &key_schedule);
for (int i = 0; i < strlen(ciphertext); i += BLOCK_SIZE) {
DES_cblock ciphertext_block, decrypted_block;
memcpy(ciphertext_block, ciphertext + i, BLOCK_SIZE);
DES_ecb_encrypt(&ciphertext_block, &decrypted_block, &key_schedule, DES_DECRYPT);
memcpy(decrypted_text + i, decrypted_block, BLOCK_SIZE);
}
}
int main() {
// 测试
char key[] = "abcdefgh";
char plaintext[] = "hello, world!";
char encrypted_text[strlen(plaintext) + 1];
char decrypted_text[strlen(plaintext) + 1];
encrypt(key, plaintext, encrypted_text);
decrypt(key, encrypted_text, decrypted_text);
printf("加密后的文本:%s\n", encrypted_text);
printf("解密后的文本:%s\n", decrypted_text);
return 0;
}
```
需要安装 OpenSSL 库来使用 DES 加密和解密。以上代码中,我们使用了基本的 ECB 模式,这是不安全的,因为它容易受到 replay 攻击和其他攻击。在实际应用中,应使用更安全的加密模式,例如 CBC 或 CTR。
des加密c语言
以下是使用 OpenSSL 库进行 DES 加密的 C 代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/des.h>
int main() {
const char* key = "01234567"; // 8 字节的密钥
const char* plaintext = "hello, world"; // 明文
const int plaintext_len = strlen(plaintext);
// 初始化密钥
DES_cblock des_key;
DES_key_schedule key_schedule;
memcpy(des_key, key, 8);
DES_set_key(&des_key, &key_schedule);
// 分配加密结果缓冲区
const int out_buf_len = plaintext_len + 8 - plaintext_len % 8;
unsigned char* out_buf = (unsigned char*)malloc(out_buf_len);
memset(out_buf, 0, out_buf_len);
// 进行加密
DES_ncbc_encrypt((unsigned char*)plaintext, out_buf, plaintext_len, &key_schedule, &des_key, DES_ENCRYPT);
// 输出加密结果
for (int i = 0; i < out_buf_len; ++i) {
printf("%02x ", out_buf[i]);
}
printf("\n");
free(out_buf);
return 0;
}
```
需要注意的是,此代码使用了 ECB(电子密码本)模式进行加密,如果需要使用其他模式(例如 CBC、CFB、OFB),需要调用相应的加密函数,例如 `DES_ncbc_encrypt()`、`DES_cfb_encrypt()`、`DES_ofb_encrypt()`。此外,此代码没有进行填充,如果需要进行填充,可以使用 OpenSSL 库中的 `PKCS5_padding()` 函数。
阅读全文
相关推荐





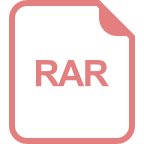
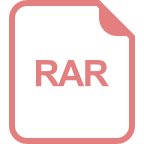
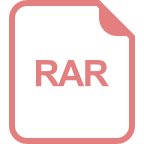

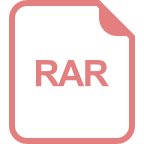



