python调用C语言实现全屏截图并传入tkimage中并在windows下显示图片
时间: 2023-06-20 15:05:36 浏览: 181
可以使用Python调用C语言的方式实现全屏截图,并将截图传入Tkinter中进行显示。下面是一个简单的实现过程:
1. 编写C语言代码实现全屏截图,这里可以使用Windows API函数实现,例如GetDesktopWindow()、GetWindowRect()和BitBlt()等函数。
```c
#include <Windows.h>
HBITMAP CaptureScreen() {
HDC hdcScreen = GetDC(NULL);
HDC hdcMem = CreateCompatibleDC(hdcScreen);
int width = GetDeviceCaps(hdcScreen, HORZRES);
int height = GetDeviceCaps(hdcScreen, VERTRES);
HBITMAP hBitmap = CreateCompatibleBitmap(hdcScreen, width, height);
HGDIOBJ hOld = SelectObject(hdcMem, hBitmap);
BitBlt(hdcMem, 0, 0, width, height, hdcScreen, 0, 0, SRCCOPY);
SelectObject(hdcMem, hOld);
DeleteDC(hdcMem);
ReleaseDC(NULL, hdcScreen);
return hBitmap;
}
```
2. 将C语言代码编译为动态链接库(DLL)文件,使用Python的ctypes模块调用DLL文件中的函数。
```c
#include <Windows.h>
__declspec(dllexport) HBITMAP CaptureScreen() {
HDC hdcScreen = GetDC(NULL);
HDC hdcMem = CreateCompatibleDC(hdcScreen);
int width = GetDeviceCaps(hdcScreen, HORZRES);
int height = GetDeviceCaps(hdcScreen, VERTRES);
HBITMAP hBitmap = CreateCompatibleBitmap(hdcScreen, width, height);
HGDIOBJ hOld = SelectObject(hdcMem, hBitmap);
BitBlt(hdcMem, 0, 0, width, height, hdcScreen, 0, 0, SRCCOPY);
SelectObject(hdcMem, hOld);
DeleteDC(hdcMem);
ReleaseDC(NULL, hdcScreen);
return hBitmap;
}
```
```python
import ctypes
from PIL import Image, ImageTk
import tkinter as tk
# 加载DLL文件
dll = ctypes.windll.LoadLibrary("screenshot.dll")
# 调用C语言函数获取全屏截图
hBitmap = dll.CaptureScreen()
# 将HBITMAP对象转换为PIL Image对象
pil_image = Image.fromhandle(hBitmap)
# 将PIL Image对象转换为Tkinter PhotoImage对象
tk_image = ImageTk.PhotoImage(image=pil_image)
# 在窗口中显示截图
root = tk.Tk()
label = tk.Label(root, image=tk_image)
label.pack()
root.mainloop()
```
注意,在使用完HBITMAP对象后需要使用DeleteObject()函数进行释放,否则会出现内存泄漏的问题。
```c
__declspec(dllexport) void DeleteBitmap(HBITMAP hBitmap) {
DeleteObject(hBitmap);
}
```
```python
# 使用完HBITMAP对象后释放内存
dll.DeleteBitmap(hBitmap)
```
阅读全文
相关推荐
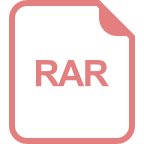
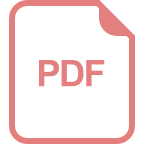
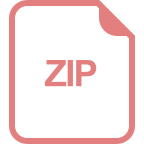
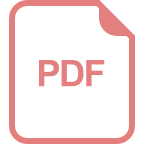
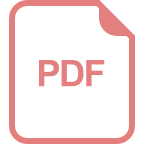
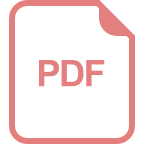
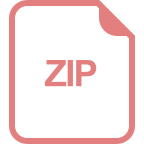
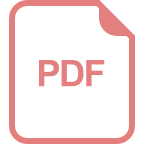
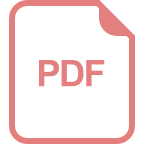
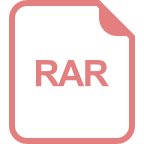
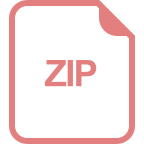
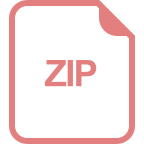
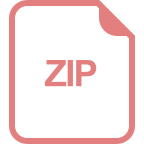
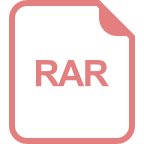
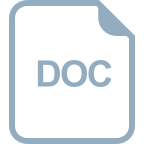
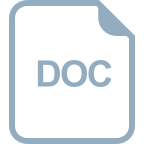