键值对(key-value pair)表中存储的是一个个键值对,如table[2016]=19.5表示该表中有一项的key为2016,其value为19.5。我们需要管理的表中, key为整数,而value可为浮点数,整数或字符。所以我们定义一个类模板,得到了三个相应的模板类。 键值对表的容量是有限的,当表中存储的key的数量达到上限时,将不存入新的键值对,但对表中已有的key仍然会继续更新其value。每行输入有三项,第一项是类型(1为浮点数,2为整数,3为字符),第二项是key,第三项是value。在处理完每行输入后,如果输入的类型信息合法,则会输出当前该类型的键值表中最小的value及其key。对于整数和字符表,若干个键值对的value值可能相同,此时输出key最小的那一项。 现在要实现该类模板。 函数接口定义: template<class T> class Table 裁判测试程序样例: #include <iostream> using namespace std; /* 请在这里填写答案 */ int main() { const int SIZE=10; int key, ty; string temp; Table<double> dT(SIZE); Table<int> iT(SIZE); Table<char> cT(SIZE); iT[2016]=19; iT[999]=27; while(cin>>ty){ switch(ty) { case 1: cin>>key; cin>>dT[key]; dT.min(); break; case 2: cin>>key; cin>>iT[key]; iT.min(); break; case 3: cin>>key; cin>>cT[key]; cT.min(); break; default:getline(cin,temp); } } return 0; }
时间: 2023-07-14 22:13:42 浏览: 56
以下是一个可能的实现,包括了Table类以及其三个模板类DoubleTable、IntTable和CharTable。其中,每个模板类都继承自Table类,并重载了min函数以满足题目要求。
```c++
#include <iostream>
#include <map>
#include <algorithm>
using namespace std;
template<class T>
class Table {
protected:
map<int, T> data;
const int capacity;
public:
Table(int capacity) : capacity(capacity) {}
T& operator[](int key) {
return data[key];
}
virtual void min() const = 0;
};
template<class T>
class DoubleTable : public Table<T> {
public:
DoubleTable(int capacity) : Table<T>(capacity) {}
void min() const override {
if (this->data.empty()) {
cout << "no result" << endl;
return;
}
auto it = min_element(this->data.begin(), this->data.end(), [](const auto& a, const auto& b) {
return a.second < b.second;
});
cout << it->first << " " << it->second << endl;
}
};
template<class T>
class IntTable : public Table<T> {
public:
IntTable(int capacity) : Table<T>(capacity) {}
void min() const override {
if (this->data.empty()) {
cout << "no result" << endl;
return;
}
auto it = min_element(this->data.begin(), this->data.end(), [](const auto& a, const auto& b) {
return a.second < b.second || (a.second == b.second && a.first < b.first);
});
cout << it->first << " " << it->second << endl;
}
};
template<class T>
class CharTable : public Table<T> {
public:
CharTable(int capacity) : Table<T>(capacity) {}
void min() const override {
if (this->data.empty()) {
cout << "no result" << endl;
return;
}
auto it = min_element(this->data.begin(), this->data.end(), [](const auto& a, const auto& b) {
return a.second < b.second || (a.second == b.second && a.first < b.first);
});
cout << it->first << " " << it->second << endl;
}
};
int main() {
const int SIZE = 10;
int key, ty;
string temp;
DoubleTable<double> dT(SIZE);
IntTable<int> iT(SIZE);
CharTable<char> cT(SIZE);
iT[2016] = 19;
iT[999] = 27;
while (cin >> ty) {
switch (ty) {
case 1: cin >> key; cin >> dT[key]; dT.min(); break;
case 2: cin >> key; cin >> iT[key]; iT.min(); break;
case 3: cin >> key; cin >> cT[key]; cT.min(); break;
default: getline(cin, temp);
}
}
return 0;
}
```
相关推荐
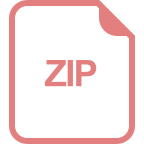
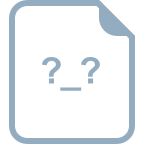
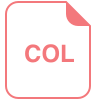
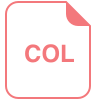
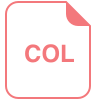
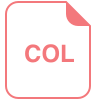
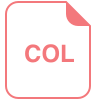










