C# 写一个http请求工具类
时间: 2023-11-22 18:36:31 浏览: 240
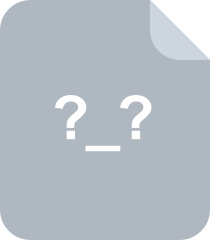
http请求工具类
可以使用 C# 自带的 HttpClient 类来实现 HTTP 请求。以下是一个简单的 HTTP 请求工具类示例:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
namespace HttpUtils
{
public class HttpUtil
{
private static readonly HttpClient client = new HttpClient();
public static async Task<string> Get(string url)
{
HttpResponseMessage response = await client.GetAsync(url);
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
public static async Task<string> Post(string url, string data)
{
HttpContent content = new StringContent(data);
HttpResponseMessage response = await client.PostAsync(url, content);
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
}
}
```
使用示例:
```csharp
using System;
using System.Threading.Tasks;
using HttpUtils;
namespace Test
{
class Program
{
static async Task Main(string[] args)
{
string result = await HttpUtil.Get("https://www.baidu.com");
Console.WriteLine(result);
result = await HttpUtil.Post("https://www.example.com/api", "{\"name\":\"John\",\"age\":30}");
Console.WriteLine(result);
}
}
}
```
阅读全文
相关推荐
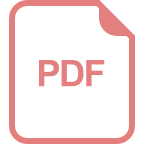
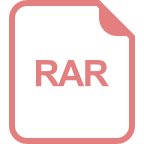
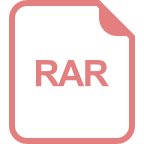
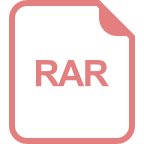
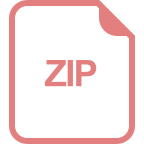
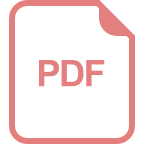
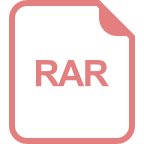
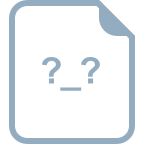
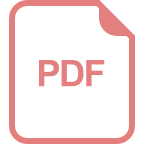
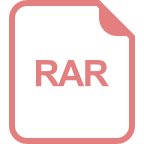
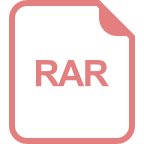
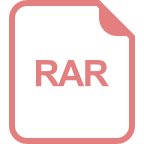