假设教师工作证包括编号、姓名、性别、出生年月、职务和签发时间;学生证包括编号、姓名、性别、出生年月、系别、入校时间。请你为教师工作证与学生工作证设计一个类结构,尽可能保证代码的重用率,并输出教师工作证和学生工作证信息。
时间: 2024-11-03 12:21:24 浏览: 3
我们可以创建两个基类,如`IdentityCard`,然后分别派生出`TeacherCertificate`和`StudentCertificate`。这样可以利用继承和封装的原则,提高代码的重用性和灵活性。以下是基础的设计:
```java
// 基础身份卡类
public abstract class IdentityCard {
private String id;
private String name;
private String gender;
private Date birthDate;
// 共享字段和构造函数
public IdentityCard(String id, String name, String gender, Date birthDate) {
this.id = id;
this.name = name;
this.gender = gender;
this.birthDate = birthDate;
}
// 获取和设置公共字段的方法
public String getId() { return id; }
public void setId(String id) { this.id = id; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public String getGender() { return gender; }
public void setGender(String gender) { this.gender = gender; }
public Date getBirthDate() { return birthDate; }
public void setBirthDate(Date birthDate) { this.birthDate = birthDate; }
// 简单的信息打印方法,供所有子类重写
public abstract String printInfo();
}
// 教师工作证类
public class TeacherCertificate extends IdentityCard {
private String position;
private Date issueTime;
public TeacherCertificate(String id, String name, String gender, Date birthDate, String position, Date issueTime) {
super(id, name, gender, birthDate);
this.position = position;
this.issueTime = issueTime;
}
@Override
public String printInfo() {
return "教师工作证:\nID: " + id + "\n姓名: " + name + "\n性别: " + gender + "\n出生日期: " + birthDate + "\n职务: " + position + "\n签发时间: " + issueTime;
}
}
// 学生证类
public class StudentCertificate extends IdentityCard {
private String department;
private Date enrollmentDate;
public StudentCertificate(String id, String name, String gender, Date birthDate, String department, Date enrollmentDate) {
super(id, name, gender, birthDate);
this.department = department;
this.enrollmentDate = enrollmentDate;
}
@Override
public String printInfo() {
return "学生证:\nID: " + id + "\n姓名: " + name + "\n性别: " + gender + "\n出生日期: " + birthDate + "\n系别: " + department + "\n入学时间: " + enrollmentDate;
}
}
```
现在,你可以创建具体的教师和学生证实例,并调用他们的`printInfo()`方法来获取详细信息。
阅读全文
相关推荐
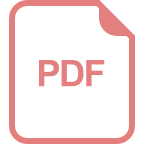
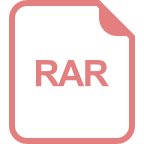


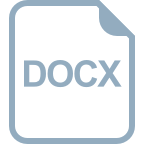
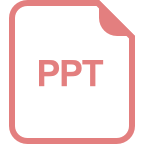
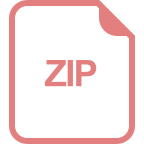
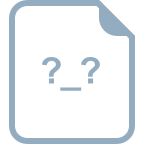
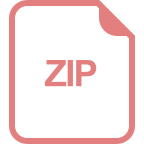
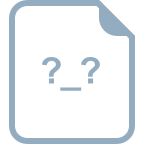
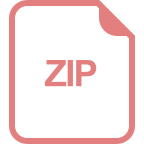
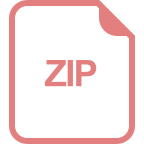