帮我用c#写一个httpclient调用post接口方法,需要入参和获取返回值,不需要异步
时间: 2024-12-02 20:28:54 浏览: 30
在C#中,你可以使用HttpClient库来发送HTTP POST请求。下面是一个简单的示例,展示了如何创建一个POST请求并传递入参,然后处理返回结果:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
public class HttpRequestManager
{
private readonly HttpClient _httpClient = new HttpClient();
// 发送POST请求的方法
public async Task<string> PostRequestAsync(string apiUrl, string requestBody)
{
try
{
// 设置Content-Type为JSON
var content = new StringContent(requestBody, Encoding.UTF8, "application/json");
// 发送POST请求
HttpResponseMessage response = await _httpClient.PostAsync(apiUrl, content);
// 检查响应状态码
if (response.IsSuccessStatusCode)
{
// 读取响应内容作为字符串
var responseBody = await response.Content.ReadAsStringAsync();
return responseBody;
}
else
{
Console.WriteLine($"Error: {response.StatusCode} - {await response.ReasonPhraseAsync()}");
return null; // 或者在这里处理错误情况
}
}
catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
return null;
}
}
// 调用方法示例
public static void Main()
{
string apiUrl = "https://example.com/api/endpoint";
string requestBody = "{\"key\":\"value\"}"; // 替换为你实际的请求体
HttpRequestManager manager = new HttpRequestManager();
string result = manager.PostRequestAsync(apiUrl, requestBody).Result; // 使用Task.Result获取同步结果
if (result != null)
{
Console.WriteLine("Response: " + result);
}
}
}
```
在这个例子中,`PostRequestAsync`方法接收API URL和请求体作为参数。注意,在`Main`函数中我们使用了`.Result`来等待任务完成,因为这是非异步的请求。如果你需要保持异步风格,可以去掉`.Result`。
阅读全文
相关推荐






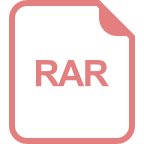
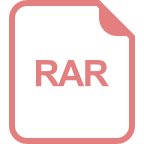










