c++用单链表建立一个班级通讯录
时间: 2024-10-13 15:06:07 浏览: 53
在 C++ 中,我们可以使用单链表数据结构来创建一个简单的班级通讯录。首先,我们需要定义一个节点(Node),它通常包含学生的信息,比如姓名、学号等,以及指向下一个节点的指针:
```cpp
struct Student {
string name;
int id;
// 如果需要,还可以添加其他属性如联系方式
Student* next; // 指向下一个学生的指针
};
// 创建一个指向节点的指针,表示链表的头
Student* classDirectory = nullptr;
```
接下来,我们可以通过以下步骤来操作这个链表作为班级通讯录:
1. **添加学生**:
```cpp
void addStudent(string name, int id) {
Student* newNode = new Student();
newNode->name = name;
newNode->id = id;
newNode->next = classDirectory;
classDirectory = newNode;
}
```
2. **查找学生**:
```cpp
bool searchStudent(int id, string& foundName) {
Student* current = classDirectory;
while (current != nullptr) {
if (current->id == id) {
foundName = current->name;
return true;
}
current = current->next;
}
foundName.clear(); // 如果未找到,则清空返回值
return false;
}
```
3. **遍历链表**(展示所有学生信息):
```cpp
void printClassDirectory() {
Student* current = classDirectory;
while (current != nullptr) {
cout << "Name: " << current->name << ", ID: " << current->id << endl;
current = current->next;
}
}
```
4. **删除学生**(如果需要的话,可以添加删除节点的操作)
阅读全文
相关推荐






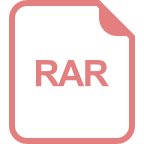










