servlet和jsp编写登录注册页面完整教程
时间: 2023-12-06 11:45:28 浏览: 150
下面是一个简单的Servlet和JSP编写登录注册页面的完整教程。
1. 创建数据库表格
首先,我们需要创建一个名为“users”的数据库表格,包含以下字段:
- id(int,自增长)
- username(varchar)
- password(varchar)
2. 创建JavaBean类
然后,我们需要创建一个JavaBean类来代表用户:
```java
public class User {
private int id;
private String username;
private String password;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
```
3. 创建数据库访问类
接下来,我们需要创建一个类来处理与数据库的交互。我们将使用JDBC连接到MySQL数据库。在这个例子中,我们将使用一个简单的DAO模式(数据访问对象)来处理所有数据库访问:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class UserDao {
private String jdbcUrl;
private String jdbcUsername;
private String jdbcPassword;
private Connection jdbcConnection;
public UserDao(String jdbcUrl, String jdbcUsername, String jdbcPassword) {
this.jdbcUrl = jdbcUrl;
this.jdbcUsername = jdbcUsername;
this.jdbcPassword = jdbcPassword;
}
protected void connect() throws SQLException {
if (jdbcConnection == null || jdbcConnection.isClosed()) {
try {
Class.forName("com.mysql.jdbc.Driver");
} catch (ClassNotFoundException e) {
throw new SQLException(e);
}
jdbcConnection = DriverManager.getConnection(jdbcUrl, jdbcUsername, jdbcPassword);
}
}
protected void disconnect() throws SQLException {
if (jdbcConnection != null && !jdbcConnection.isClosed()) {
jdbcConnection.close();
}
}
public boolean addUser(User user) throws SQLException {
String sql = "INSERT INTO users (username, password) VALUES (?, ?)";
connect();
PreparedStatement statement = jdbcConnection.prepareStatement(sql);
statement.setString(1, user.getUsername());
statement.setString(2, user.getPassword());
boolean rowInserted = statement.executeUpdate() > 0;
statement.close();
disconnect();
return rowInserted;
}
public User getUser(String username, String password) throws SQLException {
String sql = "SELECT * FROM users WHERE username = ? AND password = ?";
connect();
PreparedStatement statement = jdbcConnection.prepareStatement(sql);
statement.setString(1, username);
statement.setString(2, password);
ResultSet resultSet = statement.executeQuery();
User user = null;
if (resultSet.next()) {
user = new User();
user.setId(resultSet.getInt("id"));
user.setUsername(resultSet.getString("username"));
user.setPassword(resultSet.getString("password"));
}
resultSet.close();
statement.close();
disconnect();
return user;
}
}
```
4. 创建Servlet
现在我们可以创建一个Servlet来处理登录和注册请求。这个Servlet将使用我们刚刚创建的UserDao类来访问数据库。
```java
import java.io.IOException;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/login")
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private UserDao userDao;
public void init() {
String jdbcUrl = "jdbc:mysql://localhost:3306/test";
String jdbcUsername = "root";
String jdbcPassword = "password";
userDao = new UserDao(jdbcUrl, jdbcUsername, jdbcPassword);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
try {
User user = userDao.getUser(username, password);
if (user != null) {
request.setAttribute("user", user);
request.getRequestDispatcher("welcome.jsp").forward(request, response);
} else {
response.sendRedirect("login.jsp");
}
} catch (SQLException ex) {
throw new ServletException(ex);
}
}
}
```
5. 创建JSP
最后,我们需要创建一个JSP文件来显示登录表单和欢迎消息。
```html
<!DOCTYPE html>
<html>
<head>
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<form action="login" method="post">
<label>Username:</label>
<input type="text" name="username"><br><br>
<label>Password:</label>
<input type="password" name="password"><br><br>
<input type="submit" value="Login">
</form>
</body>
</html>
```
```html
<!DOCTYPE html>
<html>
<head>
<title>Welcome</title>
</head>
<body>
<h1>Welcome <%= request.getAttribute("user").getUsername() %>!</h1>
</body>
</html>
```
6. 部署应用程序
现在,我们已经完成了我们的应用程序。我们只需要将它部署到一个Web服务器上,例如Tomcat或Jetty,就可以开始使用它了。
注意:这只是一个简单的例子,实际的Web应用程序需要更多的安全性和错误处理。
阅读全文
相关推荐




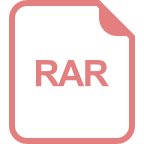





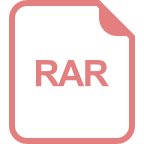
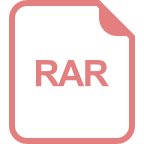
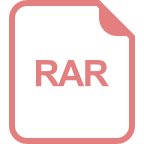
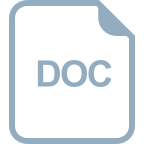




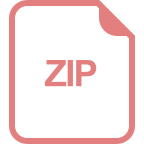