Linux中 mutex_trylock的使用方法
时间: 2024-05-14 19:15:54 浏览: 338
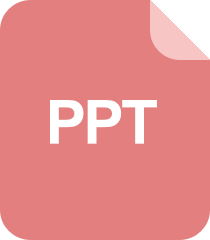
Linux 线程间同步机制
可以使用 mutex_trylock() 函数来尝试锁定一个互斥锁,该函数有以下两种返回值:
1. 如果互斥锁当前没有被锁定,则该函数会立即锁定互斥锁,并返回0。
2. 如果互斥锁当前已被锁定,则该函数不会阻塞,而是立即返回 -EBUSY 错误码。
函数定义如下:
```
int mutex_trylock(struct mutex *lock);
```
其中,参数 lock 是需要锁定的互斥锁。
用法示例:
```
#include <linux/mutex.h>
// 定义一个互斥锁
static DEFINE_MUTEX(my_mutex);
// 在某个函数中使用 mutex_trylock() 尝试锁定互斥锁
int my_function(void)
{
if (mutex_trylock(&my_mutex) == 0) {
// 互斥锁已成功锁定,可以执行相关操作
...
// 解除互斥锁
mutex_unlock(&my_mutex);
} else {
// 互斥锁已被占用,执行其他操作
...
}
return 0;
}
```
注意,mutex_trylock() 函数只是尝试锁定互斥锁,并不会阻塞等待锁定成功。如果需要阻塞等待锁定成功,则需要使用 mutex_lock() 函数。
阅读全文
相关推荐
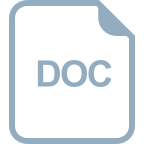
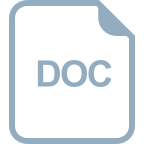










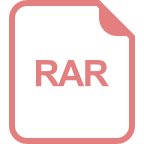
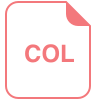


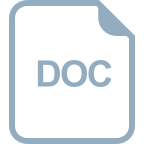
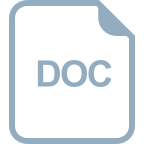