js使用replace将空格和加号替换为下划线
时间: 2023-06-03 15:07:39 浏览: 333
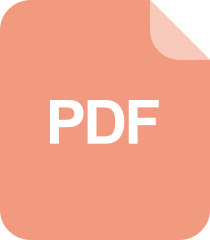
JS的replace方法
好的,我可以回答这个问题。在 JavaScript 中,可以使用 replace() 方法来替换字符串中的某些字符。要将空格和加号替换为下划线,可以使用以下代码:
```
let str = 'example string with spaces + and plus signs';
let newStr = str.replace(/[\s+]/g, '_');
console.log(newStr); // 输出: 'example_string_with_spaces___and_plus_signs'
```
这段代码中,我们使用正则表达式 /[\s+]/g 来匹配所有的空格和加号,并使用 replace() 方法将它们替换为下划线。
阅读全文
相关推荐
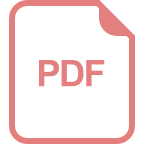
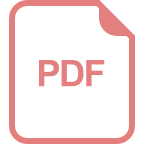
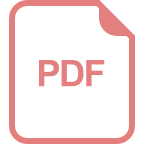
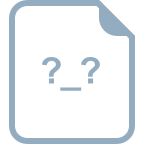
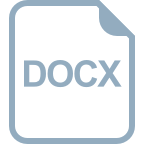
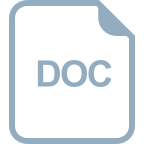
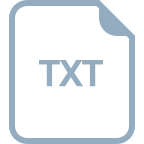
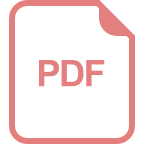
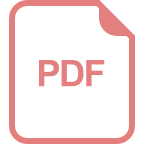
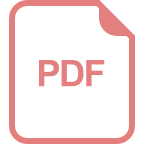
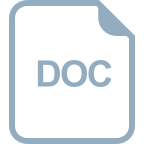
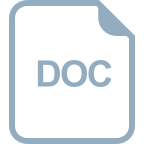
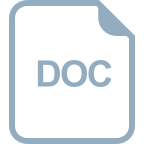
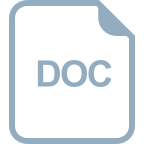
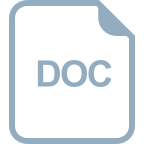
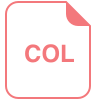
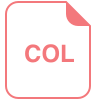