C++使用sqlite返回CString
时间: 2024-04-30 17:19:24 浏览: 7
你可以通过以下代码将 SQLite 返回的字符串转换为 CString:
```c++
// SQLite 返回的字符串
char* sqliteString = ...;
// 获取字符串长度
int nLength = strlen(sqliteString);
// 创建 CString 对象
CString csString(sqliteString, nLength);
// 释放 SQLite 返回的字符串
sqlite3_free(sqliteString);
```
注意,在使用完 SQLite 返回的字符串后,需要使用 `sqlite3_free()` 函数释放内存。否则,可能会导致内存泄漏。
相关问题
C++ 使用sqlite3
SQLite3是一款轻量级的关系型数据库管理系统,它是以C语言写成的,并且提供了C语言的API接口。要在C++中使用SQLite3,可以使用SQLite C/C++接口,该接口包含在SQLite3的源代码中,可以通过下载SQLite3的源码来获得。
以下是在C++中使用SQLite3的基本步骤:
1. 下载SQLite3的源代码并解压缩。
2. 在C++项目中添加SQLite3的源代码文件。
3. 在需要使用SQLite3的地方包含SQLite3的头文件:
```c++
#include <sqlite3.h>
```
4. 打开数据库连接:
```c++
sqlite3* db;
int rc = sqlite3_open("database.db", &db);
if(rc != SQLITE_OK) {
// 连接失败
}
```
这里的`database.db`是数据库文件的名称,如果该文件不存在,则会自动创建。
5. 执行SQL语句:
```c++
char* errmsg;
int rc = sqlite3_exec(db, "CREATE TABLE IF NOT EXISTS users(id INTEGER PRIMARY KEY, name TEXT, age INTEGER)", nullptr, nullptr, &errmsg);
if(rc != SQLITE_OK) {
// 执行SQL语句失败
}
```
上面的SQL语句创建了一个名为`users`的表,该表包含三个字段:`id`、`name`和`age`。
6. 查询数据:
```c++
sqlite3_stmt* stmt;
int rc = sqlite3_prepare_v2(db, "SELECT * FROM users", -1, &stmt, nullptr);
if(rc != SQLITE_OK) {
// 查询失败
}
while(sqlite3_step(stmt) == SQLITE_ROW) {
int id = sqlite3_column_int(stmt, 0);
const char* name = (const char*)sqlite3_column_text(stmt, 1);
int age = sqlite3_column_int(stmt, 2);
// 处理查询结果
}
sqlite3_finalize(stmt);
```
上面的代码查询了`users`表中的所有数据,并逐行读取数据。`sqlite3_prepare_v2`函数用于准备SQL语句,`sqlite3_step`函数用于执行SQL语句并读取结果,`sqlite3_finalize`函数用于释放资源。
7. 关闭数据库连接:
```c++
sqlite3_close(db);
```
关闭数据库连接会释放所有相关资源,包括打开的文件句柄、内存等。
SQLite3的API接口非常简单,使用起来也比较方便,但需要注意的是,SQLite3不支持并发访问,因此在多线程环境中使用时需要注意线程安全问题。
c++ sqlite3的使用
C++中使用SQLite3数据库可以通过SQLite3的C接口来实现。下面是一个简单的示例代码,演示了如何在C++中使用SQLite3进行数据库操作:
```cpp
#include <iostream>
#include <sqlite3.h>
int main() {
sqlite3* db;
int rc = sqlite3_open("test.db", &db);
if (rc) {
std::cerr << "Cannot open database: " << sqlite3_errmsg(db) << std::endl;
return rc;
}
char* errMsg;
rc = sqlite3_exec(db, "CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT, age INTEGER);", nullptr, nullptr, &errMsg);
if (rc != SQLITE_OK) {
std::cerr << "SQL error: " << errMsg << std::endl;
sqlite3_free(errMsg);
return rc;
}
rc = sqlite3_exec(db, "INSERT INTO users (name, age) VALUES ('John', 25);", nullptr, nullptr, &errMsg);
if (rc != SQLITE_OK) {
std::cerr << "SQL error: " << errMsg << std::endl; sqlite3_free(errMsg);
return rc;
}
sqlite3_stmt* stmt;
rc = sqlite3_prepare_v2(db, "SELECT * FROM users;", -1, &stmt, nullptr);
if (rc != SQLITE_OK) {
std::cerr << "SQL error: " << sqlite3_errmsg(db) << std::endl;
return rc;
}
while (sqlite3_step(stmt) == SQLITE_ROW) {
int id = sqlite3_column_int(stmt, 0);
const unsigned char* name = sqlite3_column_text(stmt, 1);
int age = sqlite3_column_int(stmt, 2);
std::cout << "ID: " << id << ", Name: " << name << ", Age: " << age << std::endl;
}
sqlite3_finalize(stmt);
sqlite3_close(db);
return 0;
}
```
上述代码首先打开名为"test.db"的数据库文件,如果文件不存在则会创建一个新的数据库文件。然后创建一个名为"users"的表,表中包含id、name和age三个字段。接着插入一条记录,然后查询并输出所有记录的内容。
需要注意的是,上述代码只是一个简单的示例,实际使用时可能需要更多的错误处理和安全性检查。
相关推荐
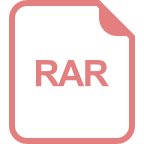
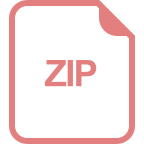
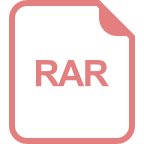












