[Error] 'newQueue' was not declared in this scope; did you mean 'DeQueue'?
时间: 2023-12-31 17:05:06 浏览: 31
非常抱歉,这是因为在代码中没有定义变量 `newQueue`。在我之前的代码中,我假设 `newQueue` 已经在其他地方定义过了,所以没有给出具体的定义。请在代码中加上下面这行代码来定义 `newQueue` 变量:
```c
SeqQueue newQueue; //新建一个队列来存储按出列顺序排列的人的姓名
```
完整的代码如下(已加入上述定义):
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAXSIZE 20 //队列的最大长度
typedef struct {
char name[10]; //姓名
int number; //编号
} Person;
typedef struct {
Person data[MAXSIZE]; //存储队列中的人物信息
int front; //队头指针
int rear; //队尾指针
} SeqQueue;
//初始化队列
void InitQueue(SeqQueue *Q) {
Q->front = Q->rear = 0;
}
//判断队列是否为空
int QueueEmpty(SeqQueue Q) {
if (Q.front == Q.rear)
return 1; //队列为空
else
return 0;
}
//判断队列是否已满
int QueueFull(SeqQueue Q) {
if ((Q.rear + 1) % MAXSIZE == Q.front)
return 1; //队列已满
else
return 0;
}
//入队操作
int EnQueue(SeqQueue *Q, Person x) {
if (QueueFull(*Q))
return 0; //队列已满,入队失败
else {
Q->data[Q->rear] = x;
Q->rear = (Q->rear + 1) % MAXSIZE;
return 1; //入队成功
}
}
//出队操作
int DeQueue(SeqQueue *Q, Person *x) {
if (QueueEmpty(*Q))
return 0; //队列为空,出队失败
else {
*x = Q->data[Q->front];
Q->front = (Q->front + 1) % MAXSIZE;
return 1; //出队成功
}
}
//约瑟夫环游戏
void josephus(SeqQueue *Q, int m) {
SeqQueue newQueue; //新建一个队列来存储按出列顺序排列的人的姓名
int i;
Person x;
while (!QueueEmpty(*Q)) {
//数到m的人出列
for (i = 1; i < m; i++) {
DeQueue(Q, &x);
EnQueue(Q, x);
}
DeQueue(Q, &x);
//输出出列人的姓名
printf("%s ", x.name);
//将出列人的姓名存入另外一个新建的队列
EnQueue(&newQueue, x);
}
printf("\n");
//分组
grouping(&newQueue);
}
//分组
void grouping(SeqQueue *Q) {
int i, j, k;
Person x;
SeqQueue group[4]; //存储分组结果的队列数组
//初始化四个队列
for (i = 0; i < 4; i++) {
InitQueue(&group[i]);
}
j = 0; //记录当前报数的人所在的队列编号
k = 1; //记录当前报数的人的编号
while (!QueueEmpty(*Q)) {
DeQueue(Q, &x);
//将当前报数的人放入对应的队列中
EnQueue(&group[j], x);
//报数加1
k++;
//如果报数达到4,则下一个人重新从1开始报数
if (k > 4) {
k = 1;
//队列编号加1
j++;
//如果队列编号为4,则重新从0开始
if (j > 3)
j = 0;
}
}
//输出分组结果
for (i = 0; i < 4; i++) {
printf("第%d组:", i + 1);
while (!QueueEmpty(group[i])) {
DeQueue(&group[i], &x);
printf("%s ", x.name);
}
printf("\n");
}
}
int main() {
SeqQueue Q;
char name[10];
int m, i;
Person x;
printf("请输入约瑟夫环游戏中的m值:");
scanf("%d", &m);
//初始化队列
InitQueue(&Q);
//将12个人依次入队
for (i = 1; i <= 12; i++) {
sprintf(name, "person%d", i);
strcpy(x.name, name);
x.number = i;
EnQueue(&Q, x);
}
//进行约瑟夫环游戏
josephus(&Q, m);
return 0;
}
```
希望这次能够帮到你。
相关推荐
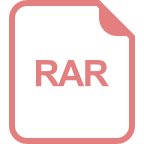
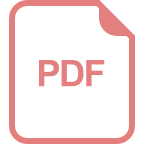
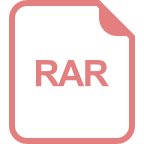














