tcp聊天程序多客户端 界面
时间: 2023-08-04 15:09:40 浏览: 81
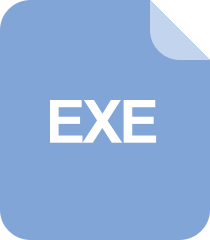
TCP多客户端程序
要实现TCP聊天程序多客户端界面,可以考虑使用图形用户界面(GUI)库,例如Python的Tkinter或PyQt等。
以下是一个简单的示例代码,可以让多个客户端通过TCP协议进行聊天:
```python
import socket
import threading
import tkinter as tk
# 创建TCP客户端
client = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 连接服务器
server_ip = '127.0.0.1'
server_port = 8888
client.connect((server_ip, server_port))
# 客户端界面
class ClientUI:
def __init__(self):
# 创建主窗口
self.root = tk.Tk()
self.root.title('TCP聊天程序')
# 创建聊天记录框
self.history = tk.Text(self.root)
self.history.pack()
# 创建消息输入框
self.message = tk.Entry(self.root)
self.message.pack()
# 创建发送按钮
self.send_button = tk.Button(self.root, text='发送', command=self.send_message)
self.send_button.pack()
# 启动接收消息的线程
threading.Thread(target=self.receive_message).start()
# 发送消息
def send_message(self):
message = self.message.get()
client.send(message.encode())
# 接收消息并显示在聊天记录框中
def receive_message(self):
while True:
message = client.recv(1024).decode()
self.history.insert(tk.END, message + '\n')
# 运行客户端界面
def run(self):
self.root.mainloop()
# 创建客户端界面
client_ui = ClientUI()
client_ui.run()
```
在这个示例代码中,我们使用了Python自带的Tkinter库来创建GUI界面,并使用socket库来实现TCP协议的通信。
启动程序后,每个客户端都会创建一个GUI界面,其中包含一个聊天记录框、一个消息输入框和一个发送按钮。当用户在消息输入框中输入消息并点击发送按钮时,程序会将消息发送给服务器。同时,程序也会启动一个线程来接收服务器发送的消息,并将消息显示在聊天记录框中。这样就可以实现多个客户端之间的聊天了。
阅读全文
相关推荐
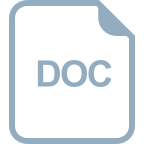
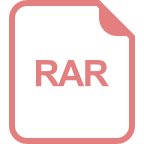
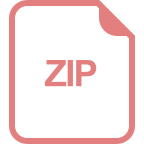
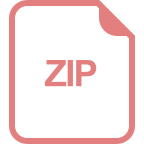
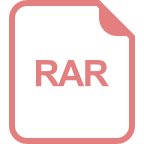
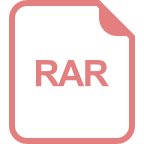
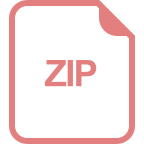
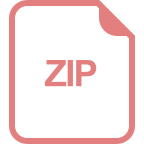
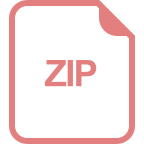
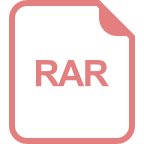
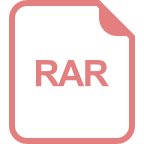
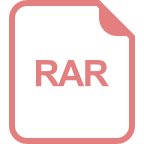
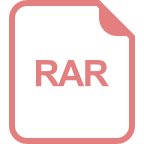
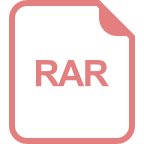
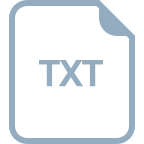
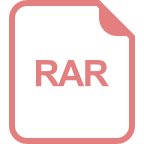