用canvas 实现烟花绽放
时间: 2023-11-16 21:54:52 浏览: 238
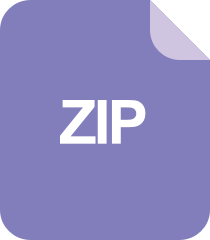
html5属性canvas实现性能堪比native体验效果的烟花播放效果.zip
要实现烟花绽放,可以按照以下步骤进行:
1. 创建一个 canvas 元素,并获取其上下文对象。
2. 定义一个烟花粒子的构造函数,包括位置、速度、颜色等属性。
3. 定义一个绘制函数,用于绘制烟花粒子。
4. 定义一个更新函数,用于更新烟花粒子的位置和速度。
5. 在每一帧中,清空画布并绘制所有烟花粒子。
6. 当某个烟花粒子达到终点时,创建一些新的烟花粒子,模拟烟花绽放的效果。
以下是示例代码:
```javascript
// 获取 canvas 上下文对象
const canvas = document.querySelector('canvas');
const ctx = canvas.getContext('2d');
// 定义烟花粒子构造函数
function Particle(x, y, vx, vy, color) {
this.x = x;
this.y = y;
this.vx = vx;
this.vy = vy;
this.color = color;
}
// 定义绘制函数
Particle.prototype.draw = function() {
ctx.beginPath();
ctx.arc(this.x, this.y, 2, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.fill();
}
// 定义更新函数
Particle.prototype.update = function() {
this.x += this.vx;
this.y += this.vy;
}
// 创建烟花粒子
function createParticle(x, y) {
const particles = [];
for (let i = 0; i < 30; i++) {
const angle = Math.random() * Math.PI * 2;
const speed = Math.random() * 5 + 2;
const vx = Math.cos(angle) * speed;
const vy = Math.sin(angle) * speed;
const color = `rgb(${Math.random() * 255}, ${Math.random() * 255}, ${Math.random() * 255})`;
particles.push(new Particle(x, y, vx, vy, color));
}
return particles;
}
// 存储所有烟花粒子
const particles = [];
// 动画循环
function loop() {
// 清空画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 绘制所有烟花粒子
particles.forEach(particle => {
particle.draw();
particle.update();
});
// 移除已经到达终点的烟花粒子
for (let i = particles.length - 1; i >= 0; i--) {
if (particles[i].y < 0) {
particles.splice(i, 1);
}
}
// 创建新的烟花粒子
if (Math.random() < 0.05) {
const x = Math.random() * canvas.width;
const y = canvas.height;
particles.push(...createParticle(x, y));
}
requestAnimationFrame(loop);
}
loop();
```
阅读全文
相关推荐
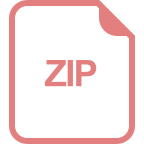
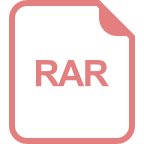
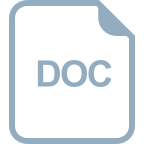
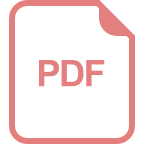
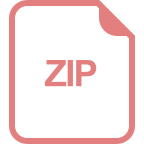
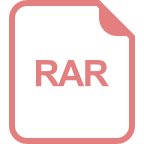
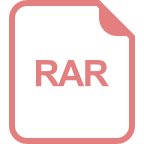
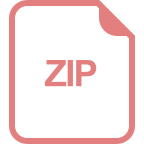
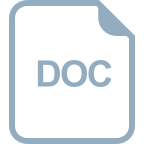
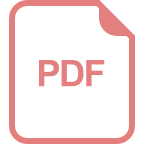
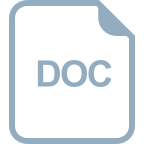
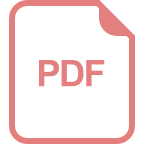
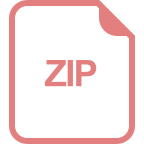